How to Print Multiple Arguments in Python
- Requirement
- Solutions - Print Multiple Arguments in Python
- Python 3.6 Only Method - F-String Formatting
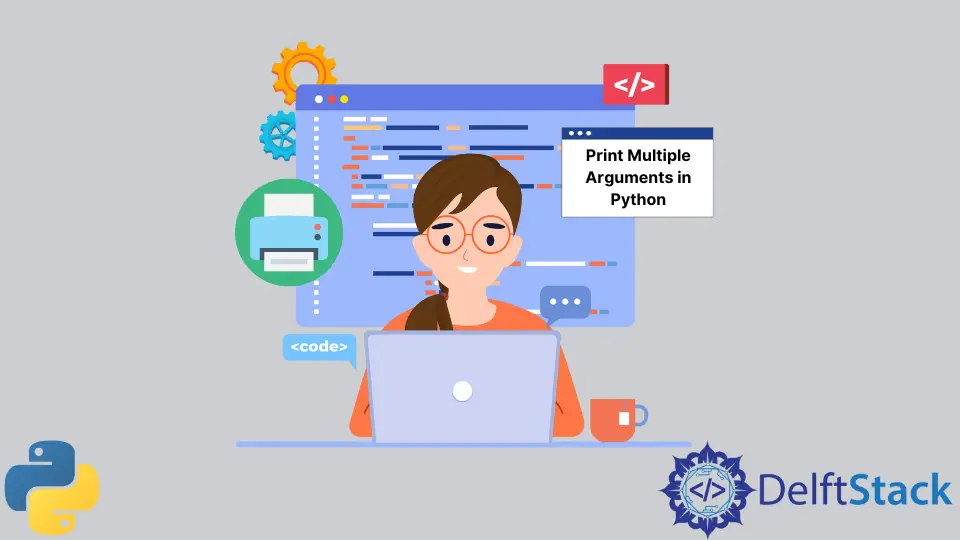
We will show you how to print multiple arguments in Python 2 and 3.
Requirement
Suppose you have two variables
city = "Amsterdam"
country = "Netherlands"
Please print the string that includes both arguments city
and country
, like below
City Amsterdam is in the country Netherlands
Solutions - Print Multiple Arguments in Python
Python 2 and 3 Solutions
1. Pass Values as Parameters
# Python 2
>>> print "City", city, 'is in the country', country
# Python 3
>>> print("City", city, 'is in the country', country)
2. Use String Formatting
There are three string formatting methods that could pass arguments to the string.
- Sequential option
# Python 2
>>> print "City {} is in the country {}".format(city, country)
# Python 3
>>> print("City {} is in the country {}".format(city, country))
- Formatting with numbers
The advantages of this option compared to the last one is that you could reorder the arguments and reuse some arguments as many as possible. Check the examples below,
# Python 2
>>> print "City {1} is in the country {0}, yes, in {0}".format(country, city)
# Python 3
>>> print("City {1} is in the country {0}, yes, in {0}".format(country, city))
- Formatting with explicit names
# Python 2
>>> print "City {city} is in the country {country}".format(country=country, city=city)
# Python 3
>>> print("City {city} is in the country {country}".format(country=country, city=city))
3. Pass Arguments as a Tuple
# Python 2
>>> print "City %s is in the country %s" %(city, country)
# Python 3
>>> print("City %s is in the country %s" %(city, country))
Python 3.6 Only Method - F-String Formatting
Python introduces a new type of string literals-f-strings
from version 3.6. It is similar to the string formatting method str.format()
.
# Only from Python 3.6
>>> print(f"City {city} is in the country {country}")
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook