How to Pretty Print a JSON File in Python
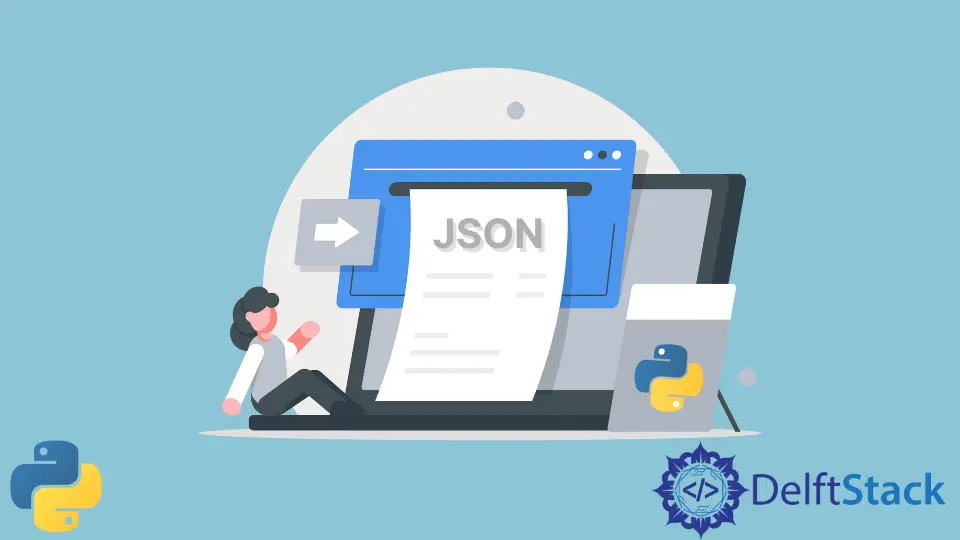
The content of JSON file could be messy if you read it to the string or load
it.
For example, in one JSON file ,
[{"foo": "Etiam", "bar": ["rhoncus", 0, "1.0"]}]
If you load
and then print
it.
import json
with open(r"C:\test\test.json", "r") as f:
json_data = json.load(f)
print(json_data)
[{"foo": "Etiam", "bar": ["rhoncus", 0, "1.0"]}]
The result is well readable compared to the standard format we normally see.
[
{
"foo": "Etiam",
"bar": [
"rhoncus",
0,
"1.0"
]
}
]
json.dumps
Method
json.dumps()
function serializes the given obj
to a JSON formatted str
.
We need to give a positive integer to the keyword parameter indent
in the json.dumps()
function to pretty print the obj
with the given indent level. If ident
is set to be 0, it will only insert new lines.
import json
with open(r"C:\test\test.json", "r") as f:
json_data = json.load(f)
print(json.dumps(json_data, indent=2))
[{"foo": "Etiam", "bar": ["rhoncus", 0, "1.0"]}]
pprint
Method
pprint
module gives the capability to pretty print Python data structures. pprint.pprint
pretty prints a Python object to a stream followed by a newline.
import json
import pprint
with open(r"C:\test\test.json", "r") as f:
json_data = f.read()
json_data = json.loads(json_data)
pprint.pprint(json_data)
The JSON file data content will be pretty printed. And you could also define the indent by assigning indent
parameter.
pprint.pprint(json_data, indent=2)
pprint
treats single '
and double quotes "
identically, but JSON
only uses "
, therefore, the pprinted
JSON
file content couldn’t be directly saved to a file.
Otherwise, the new file will not be parsed as valid JSON
format.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook