How to Get the Current Time in Python
-
Use the
datetime
Module to Get the Current Time in Python -
Use the
time
Module to Get the Current Time in Python
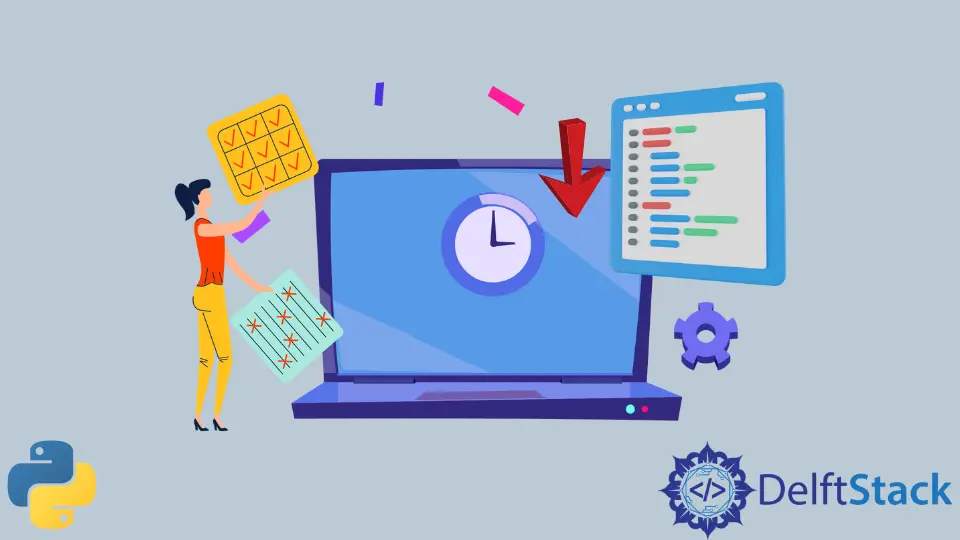
We could use two modules to get the current time in Python, that are datetime
and time
.
Use the datetime
Module to Get the Current Time in Python
>>> from datetime import datetime
>>> datetime.now()
datetime.datetime(2018, 7, 17, 22, 48, 16, 222169)
It returns the datetime
object that includes the date time information including year, month, day and time.
If you prefer a string
format, then you could use a strftime
method to convert the datetime
object instance to a string format as defined in the argument.
>>> datetime.now().strftime('%Y-%m-%d %H:%M:%S')
'2018-07-17 22:54:25'
Below is the snippet of directives in the strftime
format string.
Directive | Meaning |
---|---|
%d |
Day of the month as a decimal number [01,31]. |
%H |
Hour (24-hour clock) as a decimal number [00,23]. |
%m |
Month as a decimal number [01,12]. |
%M |
Minute as a decimal number [00,59]. |
%S |
Second as a decimal number [00,61]. |
%Y |
Year with century as a decimal number. |
Only Current Time Without Date
>>> from datetime import datetime
>>> datetime.now().time()
datetime.time(23, 4, 0, 13713)
Use the time
Module to Get the Current Time in Python
time.strftime
to Get the Current Time in Python
import time
time.strftime("%Y-%m-%d %H:%M:%S", time.localtime())
"2018-07-17 21:06:40"
time.localtime()
returns the local time in your time zone. If UTC time is preferred, then time.gmtime()
is the right choice.time.ctime
to Get the Current Time in Python
import time
time.ctime()
"Tue Oct 29 11:21:51 2019"
The result is that ctime
is more display-friendly to display in the GUI or print in the console. It could aslo be splitted to get the weekday, month, day, time and year.
>>> import time
>>> A = time.ctime()
>>> A = A.split()
>>> A
['Tue', 'Oct', '29', '12:38:44', '2019']
time.ctime()
is operation system dependent, or in other words, it could change if the OS is different. Don’t expect it to be standard among different operation systems.This method is not good for record-keeping.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook