How to Get the Current Script File Directory in Python
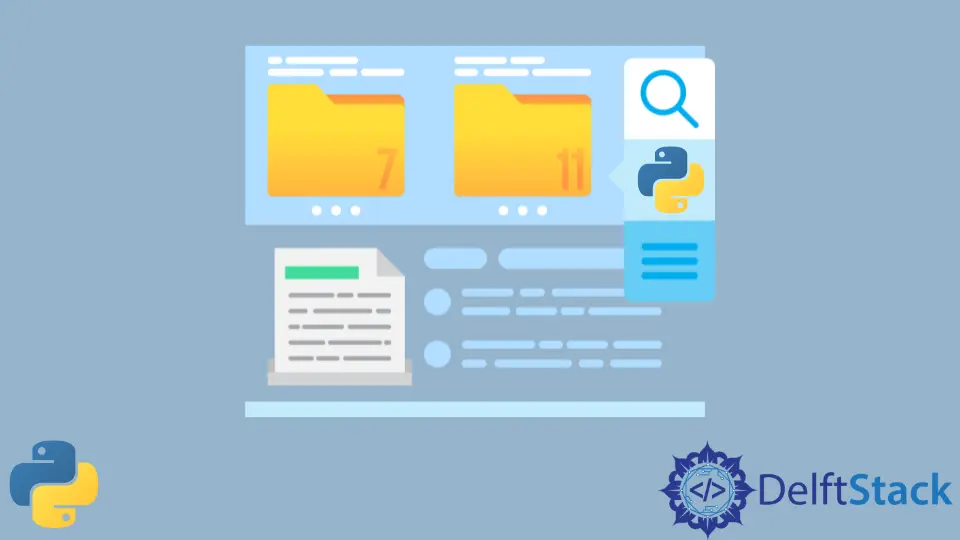
When working with Python scripts, knowing the current directory of your script file can be incredibly useful. Whether you’re managing file paths for data input/output, organizing modules, or simply navigating your project, having the correct directory path can save you a lot of headaches.
In this article, we’ll explore different methods to retrieve the current script file directory in Python. We will provide clear code examples and detailed explanations to ensure you can easily implement these techniques in your own projects. Let’s dive into the world of Python file handling and discover how to get the path of your script effortlessly.
Method 1: Using os.path
The os
module is a powerful tool in Python that allows you to interact with the operating system. One of its functionalities is to help you determine the path of the current script file. The os.path
module provides a straightforward way to get the directory of the script you are currently running. Here’s how to do it:
import os
current_directory = os.path.dirname(os.path.abspath(__file__))
print("Current Script Directory:", current_directory)
Output:
Current Script Directory: /path/to/your/script
In this example, we first import the os
module. The os.path.abspath(__file__)
retrieves the absolute path of the current script file. Then, os.path.dirname()
extracts the directory from that path. This method is reliable and works well in most environments, ensuring that you get the correct directory regardless of where your script is executed from.
Method 2: Using pathlib
If you prefer a more modern approach, Python’s pathlib
module provides an object-oriented interface to handle filesystem paths. This method is not only cleaner but also more intuitive for those who are used to working with objects. Here’s how you can use pathlib
to get the current script directory:
from pathlib import Path
current_directory = Path(__file__).resolve().parent
print("Current Script Directory:", current_directory)
Output:
Current Script Directory: /path/to/your/script
In this code snippet, we import Path
from the pathlib
module. By creating a Path
object with __file__
, we can call the resolve()
method to get the absolute path. Finally, the parent
attribute gives us the directory containing the script. This method is particularly advantageous for its readability and ease of use, making it a popular choice among Python developers.
Method 3: Using inspect
Module
Another interesting approach to find the current script directory is to use the inspect
module. This method can be particularly useful when you want to get the path of the currently executing script, especially if it’s being called from a different context. Here’s how you can do it:
import inspect
import os
current_directory = os.path.dirname(os.path.abspath(inspect.getfile(inspect.currentframe())))
print("Current Script Directory:", current_directory)
Output:
Current Script Directory: /path/to/your/script
In this example, we first import the inspect
module along with os
. The inspect.currentframe()
function retrieves the current stack frame, and inspect.getfile()
gets the file associated with that frame. Finally, we use os.path.dirname()
and os.path.abspath()
to obtain the directory path. This method is particularly useful for more complex applications where scripts may be called from various contexts, providing flexibility in path resolution.
Conclusion
Knowing how to get the current script file directory in Python is essential for effective file management and organization. Whether you choose to use the os
module, the more modern pathlib
, or the inspect
module, each method has its advantages and can be easily implemented in your projects. By understanding these techniques, you can streamline your Python workflows and avoid common pitfalls associated with file path management. So go ahead and try out these methods in your own scripts!
FAQ
-
How do I get the directory of a file that is not a script?
You can use the same methods described above but replace__file__
with the path of the file you want to investigate. -
Can I use these methods in a Jupyter Notebook?
Yes, these methods can be used in Jupyter Notebooks, but you may need to adjust the path resolution depending on how the notebook is executed. -
Is there a difference between
os.path
andpathlib
?
Yes,os.path
is a traditional way to handle paths, whilepathlib
offers an object-oriented approach that is often more intuitive and easier to read. -
What if my script is executed from a different directory?
Both methods will return the directory where the script resides, not the directory from which it was executed, ensuring you always get the correct path.
- Can I use these methods in Python 2.x?
Thepathlib
module is only available in Python 3.4 and later. However,os.path
andinspect
can be used in Python 2.x.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook