How to Generate All Permutations of a List in Python
Hassan Saeed
Feb 02, 2024
-
Use
itertools.permutations
to Generate All Permutations of a List in Python - Use Recursion to Generate All Permutations of a List in Python
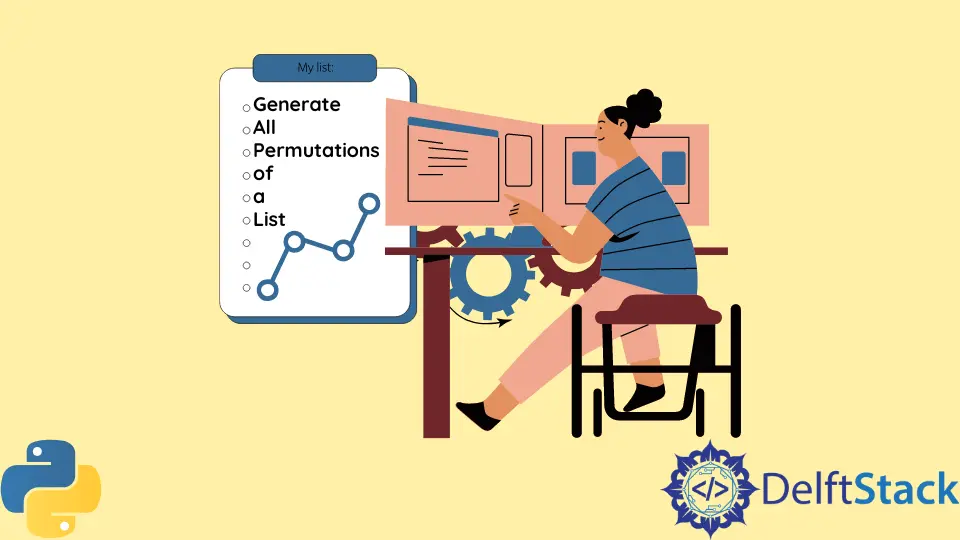
This tutorial discusses methods to generate all permutations of a list in Python.
Use itertools.permutations
to Generate All Permutations of a List in Python
Python provides a standard library tool to generate permutations: itertools.permutation
. The below example shows how to use this to generate all permutations of a list.
import itertools
inp_list = [4, 5, 6]
permutations = list(itertools.permutations(inp_list))
print(permutations)
Output:
[(4, 5, 6), (4, 6, 5), (5, 4, 6), (5, 6, 4), (6, 4, 5), (6, 5, 4)]
The default length of the permutation is set to be the length of the input list. However, we can specify the length of the permutation in the itertools.permutations
function call. The below example illustrates this.
import itertools
inp_list = [1, 2, 3]
permutations = list(itertools.permutations(inp_list, r=2))
print(permutations)
Output:
[(4, 5), (4, 6), (5, 4), (5, 6), (6, 4), (6, 5)]
The below example illustrates how to generate all permutations of all possible lengths of a given list.
import itertools
inp_list = [1, 2, 3]
permutations = []
for i in range(1, len(inp_list) + 1):
permutations.extend(list(itertools.permutations(inp_list, r=i)))
print(permutations)
Output:
[(4,), (5,), (6,), (4, 5), (4, 6), (5, 4), (5, 6), (6, 4), (6, 5), (4, 5, 6), (4, 6, 5), (5, 4, 6), (5, 6, 4), (6, 4, 5), (6, 5, 4)]
Use Recursion to Generate All Permutations of a List in Python
We can also use recursion to generate all permutations of a list in Python. The below example illustrates this.
def permutations(start, end=[]):
if len(start) == 0:
print(end)
else:
for i in range(len(start)):
permutations(start[:i] + start[i + 1 :], end + start[i : i + 1])
permutations([4, 5, 6])
Output:
[4, 5, 6]
[4, 6, 5]
[5, 4, 6]
[5, 6, 4]
[6, 4, 5]
[6, 5, 4]
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python