How to Convert String to Bytes in Python
-
bytes
Constructor to Convert String to Bytes in Python -
str.encode
Method to Convert String to Bytes in Python
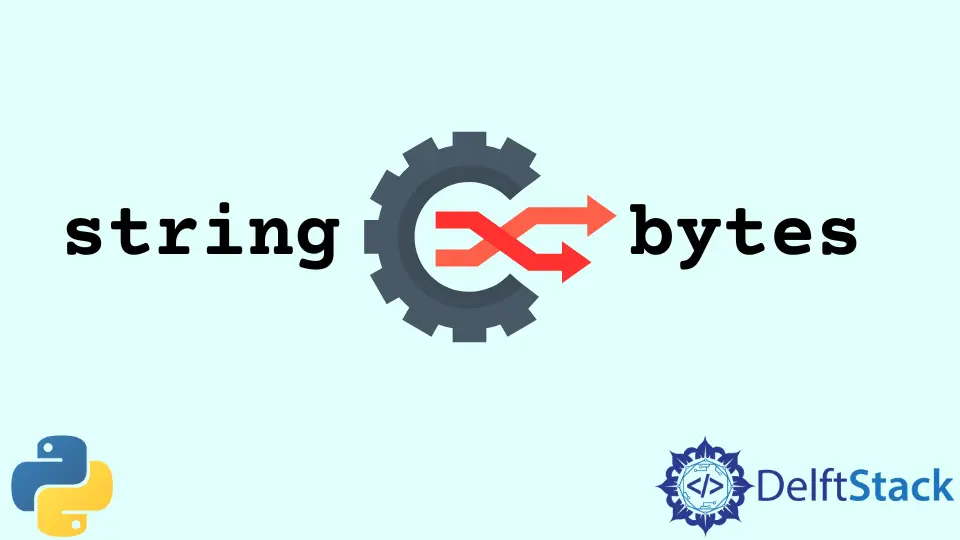
We will introduce methods to convert string to bytes in Python 3. It is the reverse operation of converting bytes
to string/howto/python/how-to-convert-bytes-to-string-in-python-2-and-python-3/.
bytes
data type is a built-in type introduced from Python 3, and bytes
in Python 2.x is actually the string
type, therefore we don’t need to introduce this conversion in Python 2.x.
bytes
Constructor to Convert String to Bytes in Python
bytes
class constructor constructs an array of bytes from data like string.
bytes(string, encoding)
We need to specify the encoding
argument, otherwise, it raises a TypeError
.
>>> bytes("Test", encoding = "utf-8")
b'Test'
>>> bytes("Test")
Traceback (most recent call last):
File "<pyshell#1>", line 1, in <module>
bytes("Test")
TypeError: string argument without an encoding
str.encode
Method to Convert String to Bytes in Python
str.encode(encoding=)
encode
method of string
class could also convert the string to bytes in Python. It has one advantage compared to the above method, that is, you don’t need to specify the encoding
if your intended encoding
is utf-8
.
>>> test = "Test"
>>> test.encode()
b'Test'
>>> test.encode(encoding="utf-8")
b'Test'
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - Python Bytes
- How to Convert Bytes to Int in Python 2.7 and 3.x
- How to Convert Int to Bytes in Python 2 and Python 3
- How to Convert Int to Binary in Python
- How to Convert Bytes to String in Python 2 and Python 3
- B in Front of String in Python