How to Convert a List to String in Python
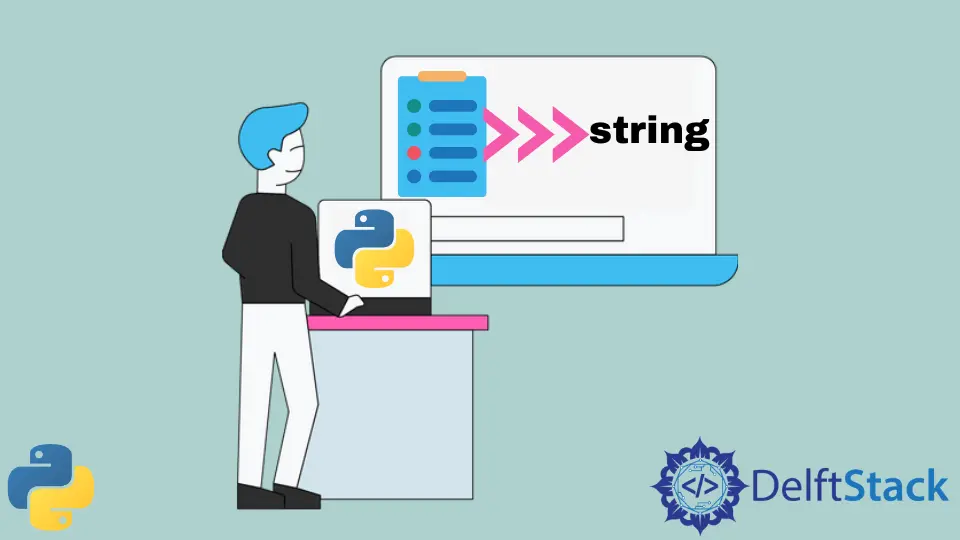
Convert a str
List to String in Python
We could use str.join()
method to convert a list that has str
data type elements to a string.
For example,
A = ["a", "b", "c"]
StrA = "".join(A)
print(StrA)
# StrA is "abc"
join
method concatenates any number of strings, the string whose method is called is inserted in between each given string. As shown in the example, the string ""
, an empty string, is inserted between the list elements.
If you want a space " "
is added between the elements, then you should use
StrA = " ".join(A)
# StrA is "a b c"
Convert a Non- str
List to a String in Python
The join
method requires the str
data type as the given parameters. Therefore, if you try to join the int
type list, you will get the TypeError
>>> a = [1,2,3]
>>> "".join(a)
Traceback (most recent call last):
File "<pyshell#1>", line 1, in <module>
"".join(a)
TypeError: sequence item 0: expected str instance, int found
The int
type should be converted to str
type before it is joined.
List comprehension
>>> a = [1,2,3]
>>> "".join([str(_) for _ in a])
"123"
map
function
>>> a = [1,2,3]
>>> "".join(map(str, a))
'123'
map
function applies the str
function to all the items in the list a
, and it returns an iterable map
object.
"".join()
iterates all the elements in the map
object and returns the concatenated elements as a string.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python