How to Convert a Character to an Integer and Vice Versa in Python
- Converting a Character to an Integer
- Converting an Integer to a Character
- Practical Applications of Character-Integer Conversions
- Conclusion
- FAQ
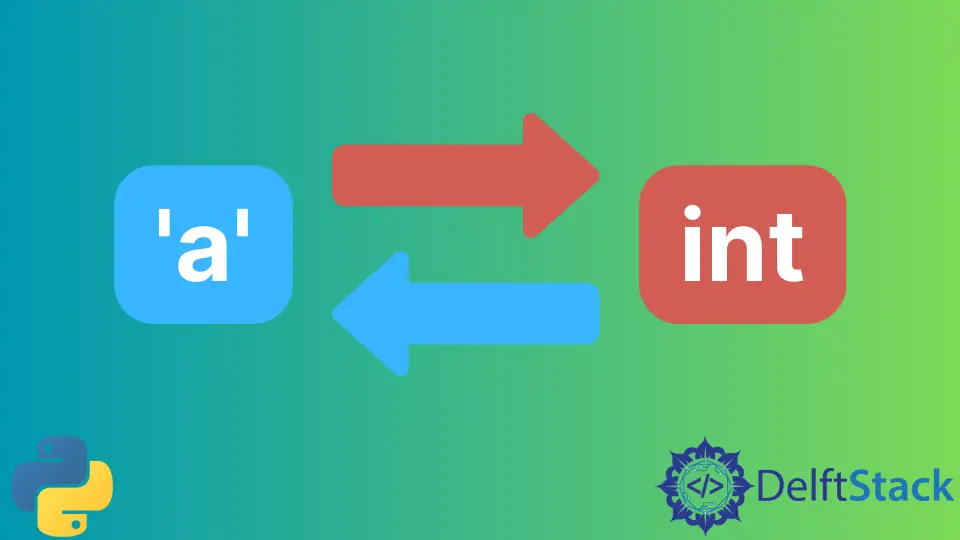
Converting characters to integers and integers to characters is a fundamental skill in Python programming. Whether you’re working on a simple script or a complex application, understanding these conversions can enhance your coding efficiency. In Python, this process is quite intuitive thanks to built-in functions.
This article will guide you through the methods to seamlessly convert characters to integers and vice versa, ensuring you have a solid grasp of these concepts. We will provide clear code examples and detailed explanations to help solidify your understanding. So, let’s dive in!
Converting a Character to an Integer
In Python, converting a character to an integer typically involves using the built-in ord()
function. This function takes a single character as an argument and returns its corresponding Unicode code point, which is an integer representation of that character.
Here’s a simple example to illustrate this:
char = 'A'
integer_value = ord(char)
print(integer_value)
Output:
65
In this example, we define a character char
with the value ‘A’. By passing this character to the ord()
function, we retrieve its Unicode code point, which is 65. This conversion is particularly useful when you need to perform operations based on the character’s numerical representation, like sorting or comparing characters.
It’s important to note that ord()
only works with a single character. If you try to pass a string with more than one character, Python will raise a TypeError
. So, when using this function, ensure that your input is strictly a single character.
Converting an Integer to a Character
Conversely, if you have an integer and want to convert it back to a character, you can use the chr()
function. This function takes an integer (representing a Unicode code point) and returns the corresponding character.
Let’s look at an example:
integer_value = 65
char = chr(integer_value)
print(char)
Output:
A
In this snippet, we start with an integer value of 65. By using the chr()
function, we convert this integer back to its corresponding character, which is ‘A’. This method is equally straightforward and allows you to easily revert from numerical representations back to their character forms.
The chr()
function is useful in scenarios where you might be processing data that requires conversion from integers to characters, such as generating sequences or handling user input. Just like with ord()
, make sure that the integer you provide is within the valid range of Unicode code points, which is typically between 0 and 1,114,111.
Practical Applications of Character-Integer Conversions
Understanding how to convert characters to integers and vice versa can be incredibly beneficial in various programming scenarios. For instance, if you are developing a game that requires user input for character selection, converting characters to their integer representations can help in implementing scoring systems or character attributes.
Moreover, these conversions are essential in data manipulation tasks. When working with strings, you may need to analyze or transform data based on character values. For example, sorting a list of characters based on their Unicode values can be efficiently handled using these conversion methods.
Here’s a practical example where we sort a list of characters based on their integer values:
chars = ['A', 'C', 'B', 'E', 'D']
sorted_chars = sorted(chars, key=ord)
print(sorted_chars)
Output:
['A', 'B', 'C', 'D', 'E']
In this code, we have a list of characters. By using the sorted()
function along with ord
as the key, we sort the characters based on their integer values. This showcases the power of converting characters to integers to facilitate operations that require ordering.
Conclusion
In conclusion, converting characters to integers and vice versa in Python is a straightforward process made easy by the built-in ord()
and chr()
functions. These conversions are not only essential for data manipulation but also play a significant role in various programming tasks. By mastering these methods, you can enhance your coding skills and apply them effectively in your projects. Whether you are sorting characters or processing user input, understanding these conversions will undoubtedly make you a more proficient Python programmer.
FAQ
-
What is the purpose of the ord() function in Python?
The ord() function in Python is used to convert a single character into its corresponding integer Unicode code point. -
Can I use ord() on a string with more than one character?
No, ord() only works with a single character. Passing a string with multiple characters will raise a TypeError. -
What does the chr() function do?
The chr() function converts an integer Unicode code point back into its corresponding character.
-
Are there any limitations to the chr() function?
Yes, the integer provided to chr() must be within the valid range of Unicode code points, typically between 0 and 1,114,111. -
How can I sort a list of characters based on their integer values?
You can use the sorted() function along with ord() as the key to sort a list of characters based on their integer representations.