How to Check Whether a String Contains Substring in Python
-
in
Operator to Check Whether a String Contains a Substring -
str.find()
Method to Check Whether a String Contains Substring -
str.index()
Method - Substring Checking Solution Conclusion
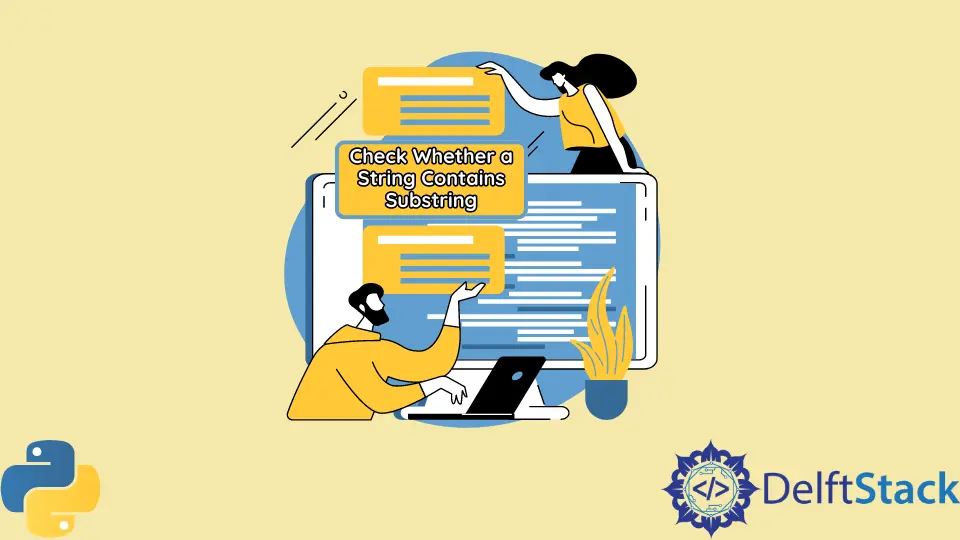
It is quite often that we need to check whether the given string contains a specific substring. We will list some methods here, and then compare the running time performance to select the most efficient method.
We will take the string - It is a given string
as the given string and given
is the substring to be checked.
in
Operator to Check Whether a String Contains a Substring
in
operator is the membership checking operator. x in y
is evaluated to be True
if x
is a member of y
, or in other words, y
contains x
.
It returns True
if the string y
contains the substring x
.
>>> "given" in "It is a given string"
True
>>> "gaven" in "It is a given string"
False
in
Operator Performance
import timeit
def in_method(given, sub):
return sub in given
print(min(timeit.repeat(lambda: in_method("It is a given string", "given"))))
0.2888628
str.find()
Method to Check Whether a String Contains Substring
find
is a built-in method of string
- str.find(sub)
.
It returns the lowest index in str
where substring sub
is found, otherwise returns -1
if sub
is not found.
>>> givenStr = 'It is a given string'
>>> givenStr.find('given')
8
>>> givenStr.find('gaven')
-1
str.find()
Method Performance
import timeit
def find_method(given, sub):
return given.find(sub)
print(min(timeit.repeat(lambda: find_method("It is a given string", "given"))))
0.42845349999999993
str.index()
Method
str.index(sub)
is a string
built-in method that returns the lowest index in str
where sub
is found. It will raise ValueError
when the substring sub
is not found.
>>> givenStr = 'It is a given string'
>>> givenStr.index('given')
8
>>> givenStr.index('gaven')
Traceback (most recent call last):
File "<pyshell#7>", line 1, in <module>
givenStr.index('gaven')
ValueError: substring not found
str.index()
Method Performance
import timeit
def find_method(given, sub):
return given.find(sub)
print(min(timeit.repeat(lambda: find_method("It is a given string", "given"))))
0.457951
Substring Checking Solution Conclusion
in
operator is the one you should use to check whether a substring exists in the given string because it is the fasteststr.find()
andstr.index()
could also be used but not the optimal because of the poor performance
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook