How to Check if a Key Exists in a Dictionary in Python
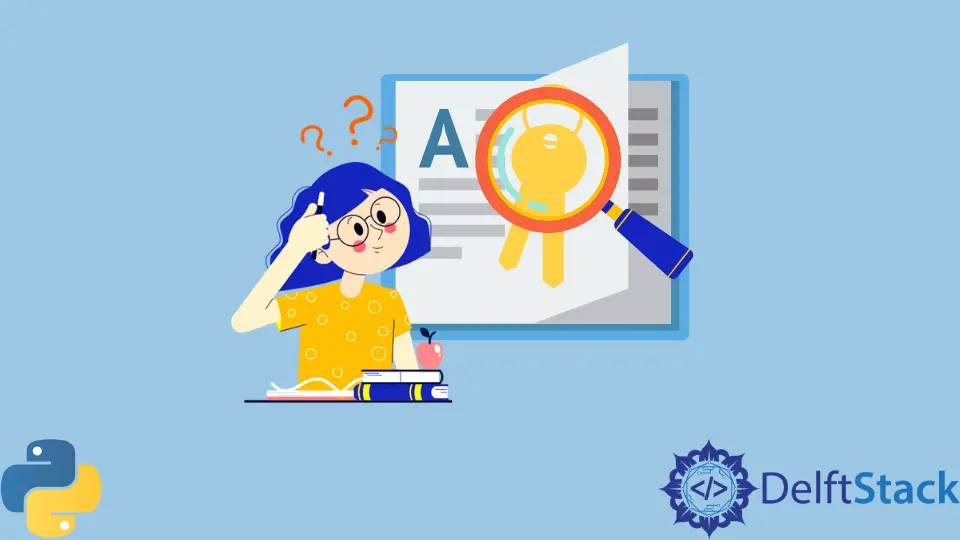
The question of how to check if a given key exists in a Python dictionary falls into the Python membership check topics that you could find more information in the tutorial here.
in
keyword is used to do the dictionary membership check. Refer to the code example below
dic = {"A": 1, "B": 2}
def dicMemberCheck(key, dicObj):
if key in dicObj:
print("Existing key")
else:
print("Not existing")
dicMemberCheck("A")
dicMemberCheck("C")
Existing key
Not existing
You could maybe have other solutions to check the given key exists in the dictionary or not, like,
if key in dicObj.keys()
It could give you the same result as the solution we just showed you. But this dicObj.keys()
method is roughly four times slower because it takes extra time to convert the dictionary keys to a list.
You could refer to the execution time performance comparison test below.
>>> import timeit
>>> timeit.timeit('"A" in dic', setup='dic = {"A":1, "B":2}',number=1000000)
0.053480884567733256
>>> timeit.timeit('"A" in dic.keys()', setup='dic = {"A":1, "B":2}',number=1000000)
0.21542178873681905
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook