How to Check a String Is Empty in a Pythonic Way
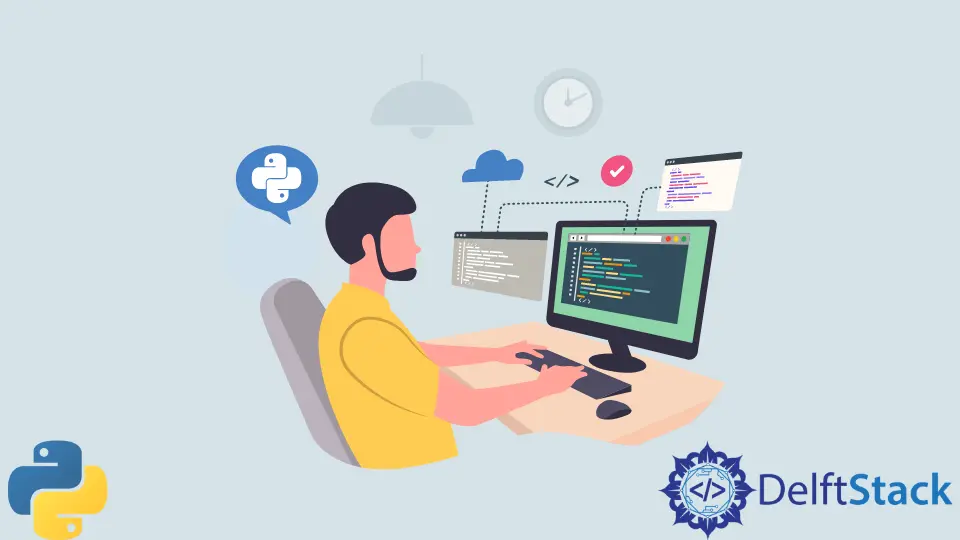
You have different methods to check whether a string is an empty string in Python. Like,
>>> A = ""
>>> A == ""
True
>>> A is ""
True
>>> not A
True
The last method not A
is a Pythonic way recommended by Programming Recommendations in PEP8. By default, empty sequences and collections are evaluated as False
in a Boolean
context.
not A
is recommended not only because it is Pythonic, but also because it is the most efficient.
>>> timeit.timeit('A == ""', setup='A=""',number=10000000)
0.4620500060611903
>>> timeit.timeit('A is ""', setup='A=""',number=10000000)
0.36170379760869764
>>> timeit.timeit('not A', setup='A=""',number=10000000)
0.3231199442780053
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook