How to Check a String Contains a Number in Python
-
Python
any
Function Withstr.isdigit
to Check Whether a String Contains a Number -
Use the
map()
Function to Check Whether a String Contains a Number -
Use
re.search(r'\d')
to Check Whether a String Contains a Number
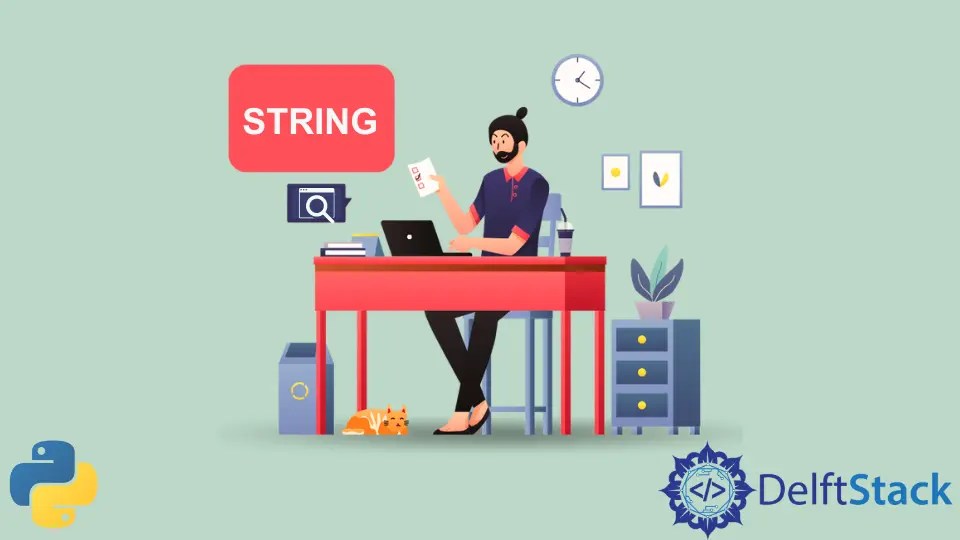
This article introduces how to check whether a Python string contains a number or not.
Python built-in any
function together with str.isdigit
will return True
if the given string contains a number in it; otherwise, it returns False
.
Python regular expression search method with pattern r'\d'
could also return True
if the given string contains a number.
Python any
Function With str.isdigit
to Check Whether a String Contains a Number
any
function returns True
if any element of the given iterable is True
; otherwise, it returns False
.
str.isdigit()
returns True
if all the characters in the given string are digits, False
otherwise.
any(chr.isdigit() for chr in stringExample)
If stringExample
contains at least a number, then the above code returns True
because chr.isdigit() for chr in stringExample
has at least one True
in the iterable.
- Working Example
str1 = "python1"
str2 = "nonumber"
str3 = "12345"
print(any(chr.isdigit() for chr in str1))
print(any(chr.isdigit() for chr in str2))
print(any(chr.isdigit() for chr in str3))
Output:
True
False
True
Use the map()
Function to Check Whether a String Contains a Number
Python map(function, iterable)
fuction applies function
to every element of the given iterable
and returns an iterator that yields the above result.
Therefore, we could rewrite the above solution to,
any(map(str.isdigit, stringExample))
- Working Example
str1 = "python1"
str2 = "nonumber"
str3 = "12345"
print(any(map(str.isdigit, str1)))
print(any(map(str.isdigit, str2)))
print(any(map(str.isdigit, str3)))
Output:
True
False
True
Use re.search(r'\d')
to Check Whether a String Contains a Number
re.search(r'\d', string)
pattern scans the string
and returns the match object for the first location that matches the given pattern - \d
that means any number between 0 and 9 and returns None
if no match is found.
import re
print(re.search(r"\d", "python3.8"))
# output: <re.Match object; span=(6, 7), match='3'>
The match
object contains the 2-tuple span
that indicates the start and end position of the match
and the matched content like match = '3'
.
bool()
function could cast the re.search
result from the match
object or None
to True
or False
.
- Working Example
import re
str1 = "python12"
str2 = "nonumber"
str3 = "12345"
print(bool(re.search(r"\d", str1)))
print(bool(re.search(r"\d", str2)))
print(bool(re.search(r"\d", str3)))
Output:
True
False
True
In terms of runtime, regular expression evaluation is much faster than using built-in string functions. If the string’s value is considerably large, then re.search()
is more optimal than using the string functions.
Compiling the expression using re.compile()
before running the search()
function on the given strings will also make the execution time even faster.
Catch the return value of compile()
into a variable and call the search()
function within that variable. In this case, search()
will only need a single parameter, that is, the string to search against the compiled expression.
def hasNumber(stringVal):
re_numbers = re.compile("\d")
return False if (re_numbers.search(stringVal) == None) else True
In summary, the built-in functions any()
and the isdigit()
can easily be used in tandem to check if a string contains a number.
However, using the utility functions search()
and compile()
within the regular expression module re
will generate results faster than the built-in string functions. So if you’re dealing with large values or strings, then the regular expression solution is much more optimal than the string functions.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook