How to Append Text to a File in Python
-
Method 1: Using the
open()
Function in Append Mode -
Method 2: Using
print()
Function with File Parameter -
Method 3: Using
pathlib
for Modern File Handling - Conclusion
- FAQ
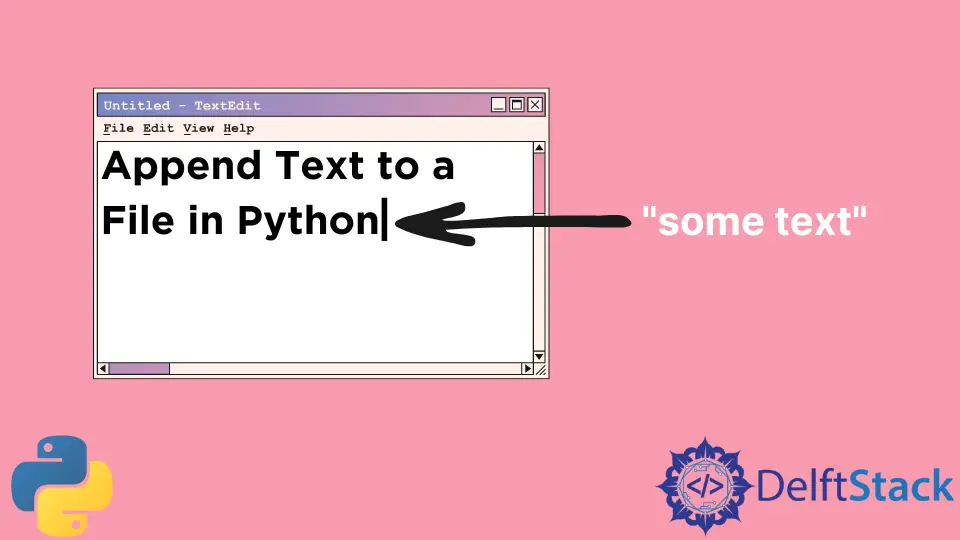
Appending text to a file in Python is a straightforward task, whether you’re using Python 2.7 or Python 3. This operation is essential for many applications, such as logging, data collection, or simply updating configuration files without overwriting existing data.
In this article, we will explore how to append text to a file using Python through various methods. We will provide clear examples and detailed explanations to help you understand each approach. Whether you’re a beginner or an experienced programmer, this guide will equip you with the knowledge to efficiently manipulate files in Python.
Method 1: Using the open()
Function in Append Mode
One of the simplest ways to append text to a file in Python is by using the built-in open()
function with the 'a'
mode. This mode opens a file for appending, allowing you to add new content without altering the existing data.
Here’s how you can do it:
with open('example.txt', 'a') as file:
file.write('This is a new line of text.\n')
Output:
This is a new line of text.
In this code snippet, we use the with
statement to open example.txt
in append mode. The with
statement ensures that the file is properly closed after its suite finishes, even if an exception is raised. Inside the block, we call file.write()
to add a line of text to the end of the file. Note that the \n
character is used to create a new line, ensuring that any subsequent text starts on a new line.
This method is efficient and straightforward, making it ideal for quick file updates. It’s also worth noting that if the file does not exist, Python will create it for you. This makes appending text a flexible option for various applications.
Method 2: Using print()
Function with File Parameter
Another effective method to append text to a file is by using the print()
function. This approach is particularly useful when you want to add formatted text or multiple items in one go.
Here’s an example:
with open('example.txt', 'a') as file:
print('This is another line of text.', file=file)
Output:
This is another line of text.
In this example, we again use the with
statement to handle the file. The print()
function has an optional file
parameter that allows you to specify the file object where the output will be directed. By passing file=file
, we direct the output of the print()
function to example.txt
, appending the specified text. This method is especially handy when you want to include multiple arguments or format your output in a specific way.
Using print()
for file output can enhance readability and make your code cleaner, especially when dealing with more complex strings or when you want to include separators between different pieces of data.
Method 3: Using pathlib
for Modern File Handling
For those using Python 3.4 and above, the pathlib
module offers an object-oriented approach to file handling. It simplifies file operations and enhances code readability. This method is particularly useful if you prefer a more modern approach to file management.
Here’s how to append text using pathlib
:
from pathlib import Path
file_path = Path('example.txt')
file_path.write_text('This is a new line of text.\n', append=True)
Output:
This is a new line of text.
In this code, we import Path
from the pathlib
module. We create a Path
object for example.txt
and then use the write_text()
method. The append=True
argument tells the method to append the new content to the file instead of overwriting it. This approach is clean and integrates well with other features of the pathlib
module, such as path manipulation and file system operations.
Using pathlib
can streamline your code, especially in larger projects where file handling is a common task. It provides a more intuitive interface and is generally preferred in modern Python programming.
Conclusion
Appending text to a file in Python is a fundamental skill that every programmer should master. Whether you choose to use the traditional open()
function, the convenient print()
method, or the modern pathlib
module, each approach has its strengths. Understanding these methods will enhance your ability to manipulate files effectively, whether for logging, data collection, or configuration updates. By practicing these techniques, you can ensure your Python scripts are both efficient and easy to maintain.
FAQ
-
How do I check if a file exists before appending text?
You can use theos.path.exists()
function to check if a file exists before appending text. -
Can I append binary data to a file in Python?
Yes, you can append binary data by opening the file in binary mode ('ab'
). -
What happens if I append text to a file that doesn’t exist?
Python will create the file for you if it does not exist when you open it in append mode. -
Is there a way to append multiple lines at once?
Yes, you can loop through a list of strings and append each line using thewrite()
method orprint()
function.
- Can I use
pathlib
to create a new file if it doesn’t exist?
Yes,pathlib
will create the file if it does not exist when you usewrite_text()
withappend=True
.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook