How to Convert Hexadecimal to Decimal in Python
-
Use the
int()
Function to Convert Hexadecimal to Integer in Python -
Use the
ast.literal_eval
Function to Convert Hexadecimal to Integer in Python
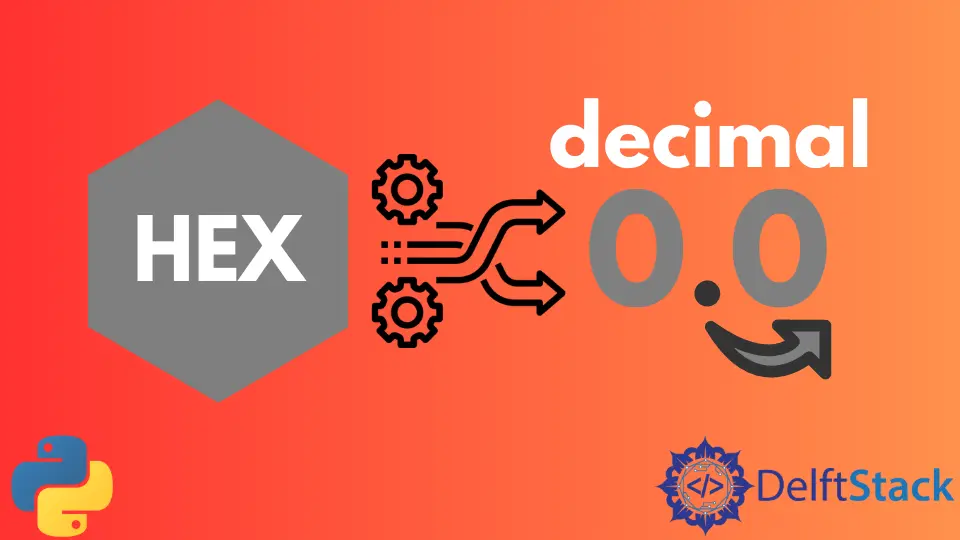
In the world of programming, we deal with values belonging to different number systems. A hexadecimal number is one such system. It is used in storing memory addresses, IP addresses, and more.
Decimal values have a base of 10, and hexadecimal values have a base of 16. In Python, hexadecimal values are prefixed with 0x
.
In this tutorial, we will learn how to convert hexadecimal values to their corresponding decimal integer numbers.
Use the int()
Function to Convert Hexadecimal to Integer in Python
The int()
function can help in converting different values to decimal integers. It typecasts the hexadecimal string to its corresponding integer value. To achieve this, we have to pass the number and its base to convert it into an integer (Remember base for hexadecimal values is 16).
s = "0xffa"
print(int(s, 16))
Output:
4090
Use the ast.literal_eval
Function to Convert Hexadecimal to Integer in Python
The ast.literal_eval()
function can evaluate string literals in Python. It can return the integer values when a hexadecimal string is passed to it, as shown below:
import ast
s = ast.literal_eval("0xffa")
print(s)
Output:
4090
Note that the eval()
function can also perform the same operation but it is recommended to use the ast.literal_eval()
. The eval()
function is very slow and is considered insecure and dangerous to use. The ast.literal_value()
also only evaluates for some of the Python datatypes, unlike the eval()
function, which evaluates for all.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn