How to Get Year From a Datetime Object in Python
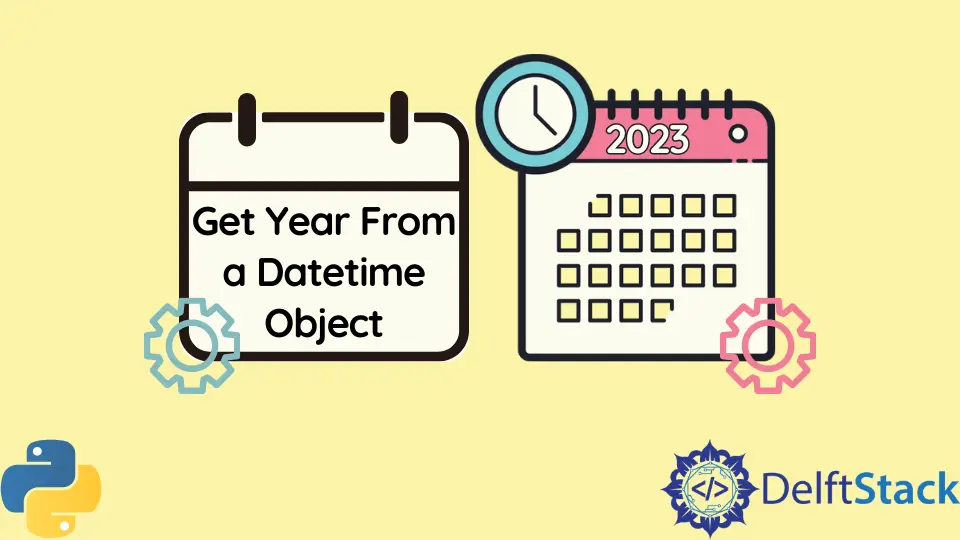
We will get the year from a datetime
object. Basically, datetime
is a module that consists of classes to deal with date and time. We can create, store, access, format the date, and much more.
Get Year From a datetime
Object in Python
The technique to get the year from a datetime
object is pretty simple. The datetime
object has certain attributes like the year, month, day, hour, minutes and seconds, etc. (depending on the format of input date).
We will access the year attribute of the datetime
object, and get the year from the date. In this example, we will get the year from today’s date (present date). For a clearer example, we output today’s date, which includes day, month, and year. Then we access the year attribute of the datetime
object and output it.
Example Code:
# python 3.x
import datetime
date = datetime.date.today()
print("Date is:", date)
year = date.year
print("Year of the Date is:", year)
Output:
Date is: 2021-12-05
Year of the Date is: 2021
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn