How to Get Web Page in Python
- Using the Requests Library
- Parsing HTML with BeautifulSoup
- Handling Errors and Exceptions
- Conclusion
- FAQ
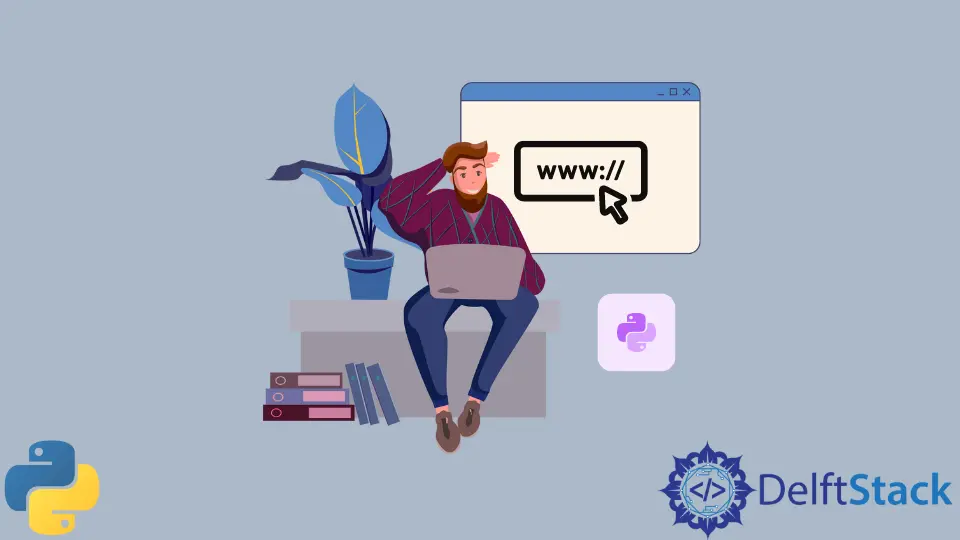
In today’s digital age, the ability to retrieve and manipulate web content programmatically is a valuable skill. Whether you’re scraping data for analysis, automating web tasks, or simply fetching information, Python provides robust libraries to help you accomplish these tasks efficiently.
This tutorial will guide you through the process of getting a web page in Python, focusing on popular methods like requests
and BeautifulSoup
. By the end of this article, you’ll have a solid understanding of how to fetch web pages and extract useful information from them. So, roll up your sleeves and let’s dive into the world of web scraping with Python!
Using the Requests Library
The requests
library is one of the most popular and user-friendly libraries for making HTTP requests in Python. It simplifies the process of sending HTTP requests and handling responses, making it an excellent choice for beginners and seasoned developers alike.
To get started, first, ensure you have the requests
library installed. You can install it using pip:
pip install requests
Once you have the library installed, you can easily fetch a webpage with a few lines of code. Here’s an example:
import requests
response = requests.get('https://www.example.com')
web_content = response.text
print(web_content)
Output:
<!DOCTYPE html>
<html>
<head>
<title>Example Domain</title>
</head>
<body>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents.</p>
</body>
</html>
In this code snippet, we begin by importing the requests
library. We then use the get()
method to send an HTTP GET request to the specified URL. The server’s response is stored in the response
variable. To access the content of the webpage, we use response.text
, which contains the HTML of the page. Finally, we print the content to the console. This method is straightforward and effective for retrieving HTML content from any accessible web page.
Parsing HTML with BeautifulSoup
Once you’ve fetched a webpage, you might want to extract specific information from it. This is where the BeautifulSoup
library comes into play. It allows you to parse HTML and XML documents and extract data from them easily.
First, make sure you have BeautifulSoup
installed. You can do this with pip as well:
pip install beautifulsoup4
Now, let’s see how to use BeautifulSoup
alongside requests
to scrape data from a webpage. Here’s a simple example:
import requests
from bs4 import BeautifulSoup
response = requests.get('https://www.example.com')
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.title.string
print(title)
Output:
Example Domain
In this example, we first import both requests
and BeautifulSoup
. After fetching the webpage content as before, we create a BeautifulSoup
object by passing the HTML content and specifying the parser (‘html.parser’). We can then easily access the title of the page using soup.title.string
, which extracts the text within the <title>
tag. This method is powerful because it allows you to navigate the HTML structure and extract any information you need, like headings, paragraphs, or links.
Handling Errors and Exceptions
When working with web requests, it’s crucial to handle potential errors and exceptions. Network issues, invalid URLs, or server errors can lead to unexpected results. By implementing error handling in your code, you can make your web scraping scripts more robust. Here’s how you can do it:
import requests
try:
response = requests.get('https://www.example.com')
response.raise_for_status()
web_content = response.text
print(web_content)
except requests.exceptions.HTTPError as err:
print(f'HTTP error occurred: {err}')
except Exception as err:
print(f'An error occurred: {err}')
Output:
<!DOCTYPE html>
<html>
<head>
<title>Example Domain</title>
</head>
<body>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents.</p>
</body>
</html>
In this code, we wrap our request in a try
block to catch exceptions. The raise_for_status()
method checks for HTTP errors, and if an error occurs, it raises an exception. We handle these exceptions with specific messages to inform the user about what went wrong. This approach ensures that your script doesn’t crash unexpectedly and provides meaningful feedback when issues arise.
Conclusion
Getting a webpage in Python is a straightforward process, thanks to libraries like requests
and BeautifulSoup
. Whether you’re simply fetching HTML content or extracting specific data, these tools provide a solid foundation for web scraping. Remember to handle errors gracefully to ensure your scripts are robust and reliable. As you continue to explore web scraping, you’ll discover a myriad of possibilities for automating tasks and gathering valuable data from the web. Happy coding!
FAQ
-
What is web scraping?
Web scraping is the process of extracting data from websites. It involves fetching web pages and parsing their content to retrieve specific information. -
Do I need permission to scrape a website?
Yes, it’s important to check a website’s terms of service and robots.txt file to understand their policies on web scraping. -
Can I use web scraping for commercial purposes?
It depends on the website’s terms of service. Always ensure you have the right to use the data you scrape, especially for commercial applications. -
Are there any alternatives to Python for web scraping?
Yes, other programming languages like JavaScript, Ruby, and PHP also offer libraries for web scraping, but Python is one of the most popular due to its simplicity and powerful libraries. -
Is web scraping legal?
The legality of web scraping varies by jurisdiction and the specific website. Always research and comply with local laws and the website’s terms of service.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn