How to Get Length of a Python Array
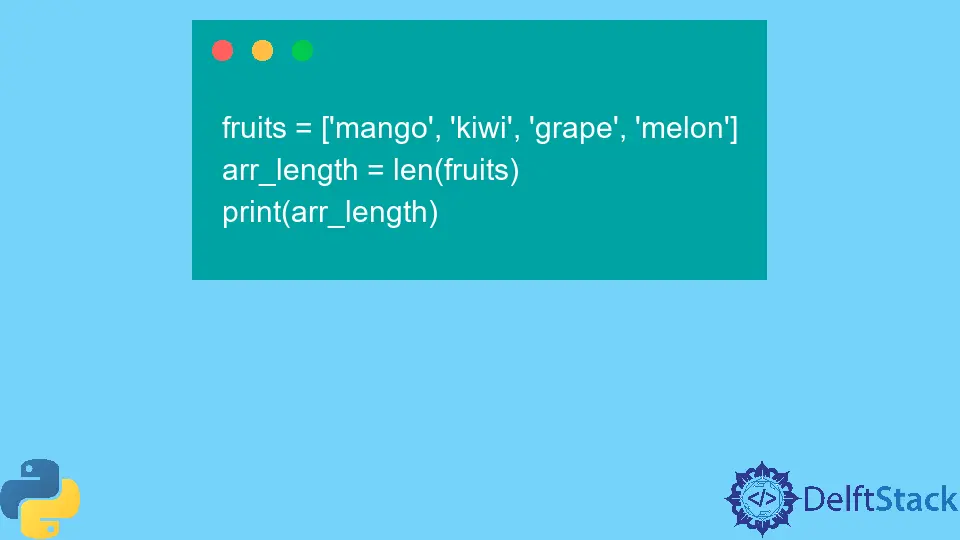
How to check an object’s length can vary from one programming language to another. While some programmers may opt to use the count()
method, others may opt for the .length()
method or the use of the .length
property to find the length.
Alternatively, Python provides the len()
function and the __len__()
method to find the length. We will look at how these two relate to each other and how the length of an object can be found using this function and method.
Using the len()
Function to Get the Length of a Python Array
len()
is a Python built-in function called on objects like tuples, queues, arrays, lists, and strings to find a total of words or characters in these objects.
__len__()
, on the other hand, is a standardized magic method (methods with two underscores before a method name and two underscores after the method name) used to return the length.
If you want to find the length of an object, call the len()
function on it by passing the object to len()
as an argument. Implicitly, Python calls the __len__()
method on the passed object to find its length. In other words, python internally translates len(object)
into object.__len__()
.
Therefore, instead of directly calling __len__()
on an object, it is preferred in Python to call the len()
function on the object. It is because of the consistency of code in Python.
When you make a call to the len()
function, you are assured of no exceptions being raised during the execution of the call, and so the program continues to run after the call. The contrary is true when the __len__()
method is used. It is uncertain that the program will not throw exceptions when the function call is run. This certainty is what we refer to as sanity checks
in Python.
We can also agree that it is more readable to call len()
on an object than it is to use __len__()
directly.
To find the length of an array, in this case, will be implemented like this:
fruits = ["mango", "kiwi", "grape", "melon"]
arr_length = len(fruits)
print(arr_length)
The example above defines an array of fruits. To find the total length of the array of fruits, define a variable that will pass the array to the len()
function. Print the variable to find the length.
Output:
4
The array returns a total of four fruits.
len()
also has a functional nature that allows it to be implemented in a different functional style like below;
length = map(len, list_of_objects)
Example:
a_list = [[1], [3, 5, 7], [2, 4, 6, 8, 10]]
print(map(len, a_list))