How to Get CPU Usage in Python
-
Use the
psutil
Library to Get the Current CPU Usage in Python -
Use the
os
Module to Retrieve Current CPU Usage in Python
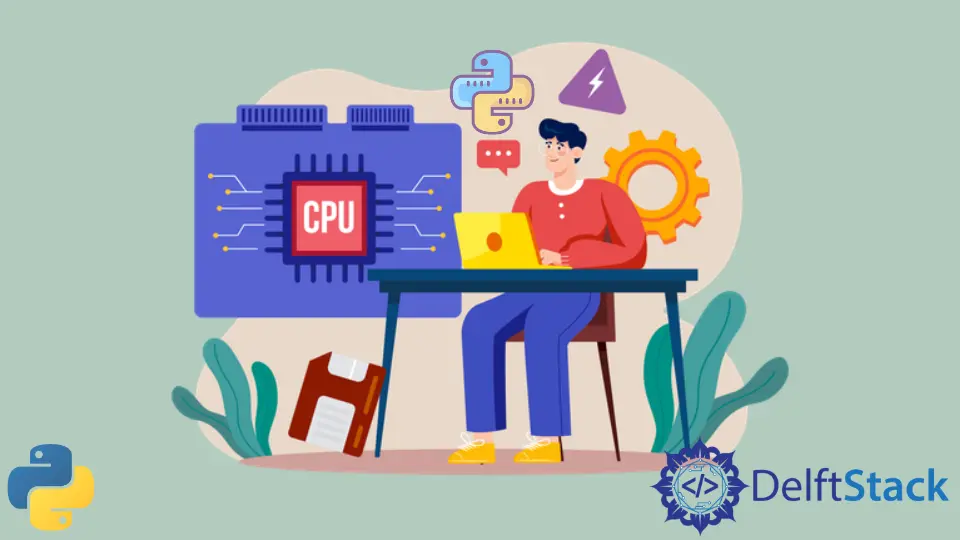
The time taken by an operating system to process some information is called its CPU usage or utilization. In this tutorial, we will get the current CPU usage of an operating system in Python.
Use the psutil
Library to Get the Current CPU Usage in Python
The psutil
module retrieves information and statistic about the system memory, CPU usage, disks, networks, and sensors. It can retrieve information from Linux, Windows, OSX, and other operating systems and is supported by most Python versions.
For example,
import psutil
print(psutil.cpu_percent())
print(psutil.cpu_stats())
print(psutil.cpu_freq())
Output:
4.6
scpustats(
ctx_switches=24804, interrupts=540172, soft_interrupts=533452226, syscalls=498969
)
scpufreq(current=2000, min=2000, max=2000)
The psutil.cpu_percent()
function is used to calculate the current CPU utilization in percent. The psutil.cpu_stats()
function gives the CPU statistics like the number of context switches, interrupts, software interrupts, and system calls. The psutil.cpu_freq()
function gives the current, minimum, and maximum CPU frequency in the unit of MHz.
Use the os
Module to Retrieve Current CPU Usage in Python
The os
module is used to interact with the operating system by providing many helpful functions. We can use the cpu_count()
function from this module to retrieve the CPU usage.
For example,
import os
import psutil
l1, l2, l3 = psutil.getloadavg()
CPU_use = (l3 / os.cpu_count()) * 100
print(CPU_use)
Output:
21.52099609375
The psutil.getloadavg()
function provides the load information about the CPU in the form of a tuple. The result obtained from this function gets updated after every five minutes. The os.cpu_count()
function gives the number of CPUs in the operating system. It returns None
if the number of CPUs is undetermined.