How to Generate GUID/UUID in Python
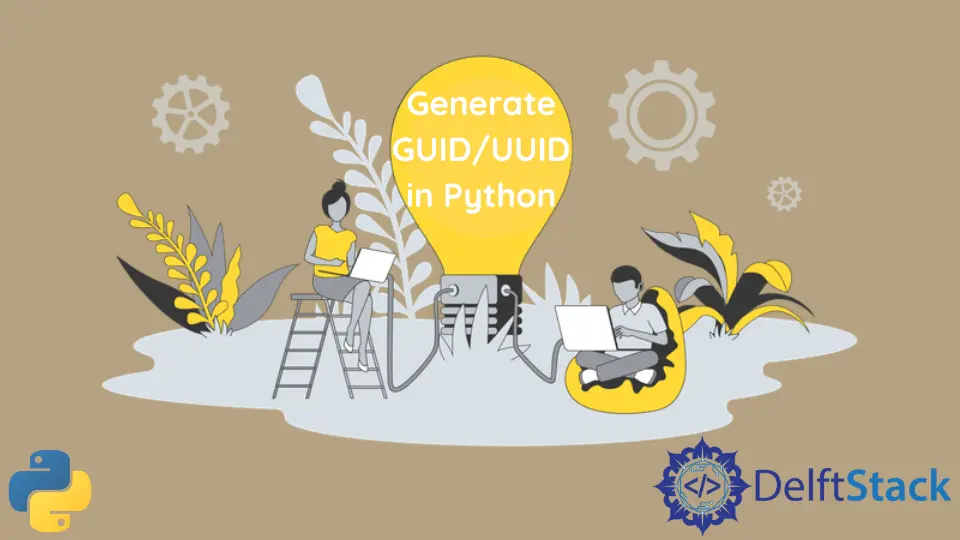
UUID is a 128-bit number used in computer systems to define entities or information uniquely. UUID stands for Universally Unique Identifier. In software created by Microsoft, UUID is regarded as a Globally Unique Identifier or GUID.
A UUID is based on two quantities: the timestamp of the system and the workstation’s unique property. This unique property could be the IP (Internet Protocol) address of the system or the MAC (Media Access Control) address.
UUIDs/GUIDs are unique in nature. Because of this property, they are widely used in software development and databases for keys.
Generate UUID/GUID in Python
To generate UUID/GUID using Python, we will use a python in-build package uuid
.
import uuid
myUUID = uuid.uuid4()
print(f"UUID/GUID -> {myUUID}")
Output:
UUID/GUID -> XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX
In the above code, the uuid4()
method generates a random UUID. The UUID returned by this function is of type uuid.UUID
. In the output, instead of XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX
, your program will output an alphanumeric string.
You can check that by running this command - print(type(uuid.uuid4()))
. It will print the following - <class 'uuid.UUID'>
You can always convert the returned UUID to string. Refer to the following code for that.
import uuid
myUUID = uuid.uuid4()
print(type(myUUID))
print(myUUID)
myUUIDString = str(myUUID)
print(type(myUUIDString))
print(myUUIDString)
Output:
<class 'uuid.UUID'>
XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX
<class 'str'>
XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX
If you wish to generate a UUID based on the current time of the machine and host ID, in that case, use the following code block.
import uuid
myUUID = uuid.uuid1()
print(f"UUID/GUID based on Host ID and Current Time -> {myUUID}")
Output:
UUID/GUID based on Host ID and Current Time ->
XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX
To learn more about uuid
, refer to the official documentation