The for...else Statement in Python
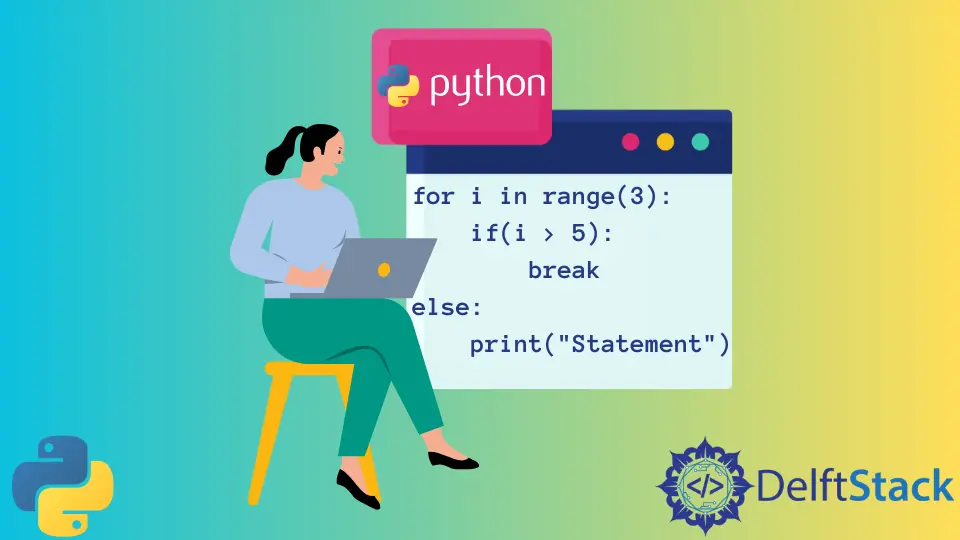
When programming in Python, you might come across the for…else statement, a unique construct that can enhance your code’s readability and functionality. While many developers are familiar with the for loop, the else clause can be a bit of a mystery.
This tutorial aims to demystify the for…else statement, providing clear examples and explanations to help you understand how to use it effectively. Whether you are a beginner or an experienced programmer looking to refine your skills, this guide will equip you with the knowledge to implement this powerful feature in your Python projects.
Understanding the for…else Statement
The for…else statement in Python is a combination of a for loop and an else clause. The else block runs after the for loop completes all its iterations, but it will not execute if the loop is terminated prematurely with a break statement. This feature can be particularly useful when searching for an item in a collection. If the item is found, you can break out of the loop; if not, the else block will execute, allowing you to handle that scenario gracefully.
Basic Structure of for…else
Here’s a simple example to illustrate the basic structure of the for…else statement:
numbers = [1, 2, 3, 4, 5]
for number in numbers:
if number == 3:
print("Found:", number)
break
else:
print("Number not found")
Output:
Found: 3
In this example, the loop iterates through the list of numbers. When it finds the number 3, it prints it and breaks out of the loop. Because the loop was exited with a break statement, the else block does not execute. If we were to search for a number that does not exist in the list, the else block would run.
Practical Use Case: Searching for an Element
Let’s explore a more practical use case where the for…else statement shines: searching for an element in a list. This example will demonstrate how the for…else construct can simplify your code when checking for the presence of an item.
fruits = ['apple', 'banana', 'cherry']
search_fruit = 'orange'
for fruit in fruits:
if fruit == search_fruit:
print(f"{search_fruit} is in the list.")
break
else:
print(f"{search_fruit} is not in the list.")
Output:
orange is not in the list.
In this case, we are searching for ‘orange’ in a list of fruits. Since ‘orange’ is not present, the for loop completes all iterations without finding a match, and the else block executes, informing us that the fruit is not in the list. This use of for…else makes the code cleaner and easier to understand compared to using a flag variable.
Advanced Example: Finding Prime Numbers
To further illustrate the versatility of the for…else statement, let’s consider an example that identifies prime numbers. This example will demonstrate how to use the for…else statement to check if a number is prime.
def is_prime(num):
if num <= 1:
return False
for i in range(2, int(num**0.5) + 1):
if num % i == 0:
print(num, "is not a prime number.")
break
else:
print(num, "is a prime number.")
is_prime(11)
is_prime(12)
Output:
11 is a prime number.
12 is not a prime number.
In this example, the function is_prime
checks if a number is prime. The for loop iterates from 2 to the square root of the number. If it finds any divisor, it prints that the number is not prime and breaks out of the loop. If the loop completes without finding a divisor, the else block executes, confirming that the number is prime. This structure allows for efficient prime checking while keeping the code organized.
Conclusion
The for…else statement in Python is a powerful tool that can enhance the clarity and efficiency of your code. By understanding how this construct works, you can handle scenarios where you need to take action based on whether a loop completed normally or was interrupted. Whether you’re searching for elements in a list or performing more complex calculations, the for…else statement can simplify your logic and make your code easier to read. So, the next time you find yourself in a situation where a loop’s completion matters, consider using the for…else statement to improve your code’s structure.
FAQ
-
What is the purpose of the else clause in a for loop?
The else clause in a for loop executes after the loop completes all iterations, unless the loop is exited with a break statement. -
Can I use for…else with other types of loops?
The for…else construct is specific to the for loop in Python. While you can use similar logic withwhile
loops, it does not have a built-in else clause. -
When should I use the for…else statement?
You should use the for…else statement when you want to handle cases where a loop completes without finding a match or when you want to execute code after all iterations have been completed. -
Is the for…else statement commonly used in Python?
While it’s not as commonly used as traditional for loops, the for…else statement can be very useful in specific scenarios, such as searching for elements in collections. -
How does the for…else statement affect performance?
The for…else statement does not inherently affect performance. However, it can make your code more readable and maintainable, which can indirectly improve development efficiency.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn