How to Fix Float Object Is Not Callable in Python
- Understanding the Float Object Is Not Callable Error
- Solution 1: Rename Your Variable
- Solution 2: Check for Reassignments
- Solution 3: Avoid Using Built-in Function Names
- Conclusion
- FAQ
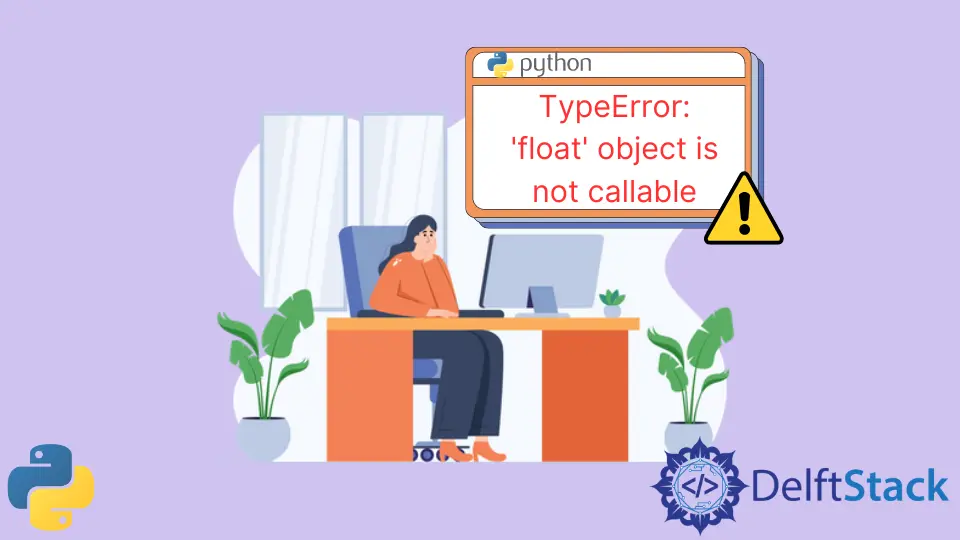
When working with Python, encountering errors can be frustrating, especially when they interrupt your workflow. One common issue is the “float object is not callable” error, which often leaves developers scratching their heads. This error typically occurs when you attempt to call a float value as if it were a function.
In this tutorial, we will explore the causes of this error and provide practical solutions to fix it. Whether you’re a beginner or an experienced programmer, understanding how to resolve this issue will enhance your coding skills and improve your Python projects. Let’s dive into the details and learn how to troubleshoot this error effectively.
Understanding the Float Object Is Not Callable Error
Before we jump into solutions, it’s essential to understand what this error means. The “float object is not callable” error occurs when you mistakenly try to use a float value like a function. For example, if you have a variable named float
that stores a float value, and later you attempt to call it as if it were a function, Python will raise this error.
Consider the following example:
float = 3.14
result = float(2)
The code above will raise an error because float
is now a float object, not the built-in float()
function.
Output:
TypeError: 'float' object is not callable
This error serves as a reminder to avoid using built-in function names for variable names. Let’s explore how to resolve this issue effectively.
Solution 1: Rename Your Variable
One of the simplest and most effective solutions to the “float object is not callable” error is to rename the variable that conflicts with the built-in float()
function. Instead of using float
as a variable name, choose a more descriptive name that reflects its purpose in your code.
Here’s an example of how to implement this solution:
my_float = 3.14
result = float(my_float)
In this code, we renamed the variable from float
to my_float
. This change allows us to call the built-in float()
function without any conflicts.
Output:
3.14
By renaming the variable, you prevent the naming conflict that caused the error. It’s a straightforward fix that can save you a lot of debugging time. This approach not only resolves the immediate issue but also enhances the readability of your code by using meaningful variable names.
Solution 2: Check for Reassignments
Another common reason for encountering the “float object is not callable” error is reassigning built-in function names. If you’ve accidentally assigned a value to a built-in function name, you’ll face this issue whenever you try to use that function.
To fix this, you need to check your code for any unintended reassignments. Here’s an example:
def calculate_area(radius):
return 3.14 * radius * radius
float = 3.14
area = calculate_area(5)
In this code, we mistakenly assigned a float value to float
, which is a built-in function in Python. This will lead to an error when you try to use float()
later in your code.
To resolve this, simply rename the variable:
pi_value = 3.14
area = calculate_area(5)
Output:
78.5
By renaming the variable from float
to pi_value
, you restore access to the built-in float()
function, allowing your code to run smoothly. Always be cautious of variable names that may conflict with built-in functions to avoid this error in the future.
Solution 3: Avoid Using Built-in Function Names
The best practice to prevent the “float object is not callable” error is to avoid using built-in function names altogether. Python has a set of reserved keywords and built-in functions that should not be used as variable names. By adhering to this guideline, you can avoid potential conflicts and errors in your code.
For instance, instead of naming your variable float
, consider using names that describe the data more accurately, such as temperature
or height
. Here’s an example:
temperature = 36.6
result = float(temperature)
Output:
36.6
By choosing a meaningful name, you not only prevent naming conflicts but also improve the clarity of your code. This practice is especially important in larger projects where readability and maintainability are crucial.
Conclusion
In summary, the “float object is not callable” error in Python is typically caused by naming conflicts with built-in functions. By renaming your variables, checking for unintended reassignments, and avoiding the use of built-in function names, you can effectively resolve this issue. Understanding the root causes of this error will not only help you fix it but also enhance your programming skills in Python. Keep these solutions in mind as you continue your coding journey, and you’ll find that troubleshooting becomes much easier over time.
FAQ
-
What does the “float object is not callable” error mean?
This error occurs when you try to call a float value as if it were a function, usually due to a naming conflict. -
How can I avoid this error in my code?
To avoid this error, do not use built-in function names, such asfloat
, as variable names. -
Can I use built-in function names as variables if I redefine them?
While you can technically redefine built-in function names, it leads to confusion and errors. It’s best to avoid this practice. -
What should I do if I encounter this error in a large codebase?
In a large codebase, search for any instances where built-in function names are used as variable names and rename them to prevent conflicts. -
Is there a way to check for naming conflicts in my code?
You can use tools like linters or IDE features that highlight naming conflicts and potential issues in your code.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Float
- How to Find Maximum Float Value in Python
- How to Convert a String to a Float Value in Python
- How to Check if a String Is a Number in Python
- How to Convert String to Decimal in Python
- How to Convert List to Float in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python