First Class Functions in Python
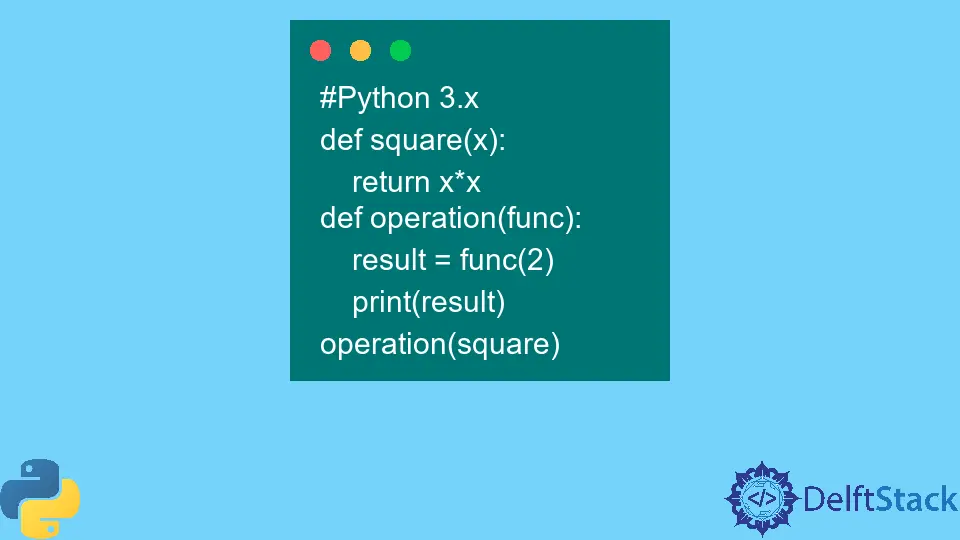
First class functions are the functions that are treated as objects or variables by the language. We can assign them to a variable or pass them as an object to some other function.
Python supports First class functions.
Properties of a First Class Function in Python
- It is an instance of the object type.
- We can assign it to a variable.
- We can pass it as an argument to another function.
- It can return a function.
- We can store it in hash tables, lists, and other data structures.
Examples of First Class Functions in Python
Functions as Objects
We assigned the function square()
to a variable my_sq
in the following code. Now, my_sq
is the same as square()
.
We didn’t call the function when we wrote its name while assigning it to the variable. We call the function my_sq()
, which refers to the actual function.
As an output, we get the square of the number passed.
Example code:
# Python 3.x
def square(x):
return x * x
my_sq = square
result = my_sq(2)
print(result)
Output:
#Python 3.x
4
Pass a Function as an Argument to Another Function
In the following code, we passed the function square()
as an argument to function operation()
just like we pass objects as arguments.
In that method, we assigned the passed function to the object func
, and we call the function square()
by calling func()
because func()
refers to square()
on the back end.
The function that accepts a function as an argument is called a higher-order function. Here, operation()
is a higher-order function.
Example code:
# Python 3.x
def square(x):
return x * x
def operation(func):
result = func(2)
print(result)
operation(square)
Output:
#Python 3.x
4
Return a Function From a Function
We can return a function from another since functions are objects. In the following code, we created a function operation()
that returns a function square()
.
We saved the returned function in an object sq
. Now, sq
refers to the method square()
.
Finally, we called the method square()
through sq
.
Example code:
# Python 3.x
def operation():
def square(x):
return x * x
return square
sq = operation()
result = sq(2)
print(result)
Output:
#Python 3.x
4
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn