How to Find Peaks in Python
- Method 1: Using NumPy for Peak Detection
- Method 2: Using SciPy for Advanced Peak Detection
- Method 3: Custom Peak Detection Algorithm
- Conclusion
- FAQ
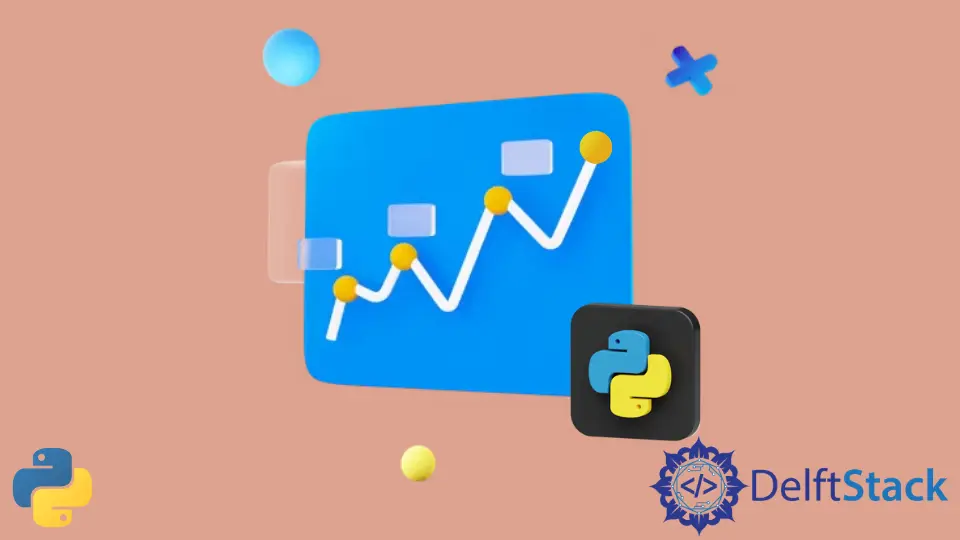
Finding peaks in data is a crucial task in many fields, from signal processing to data analysis. Peaks represent local maxima, and identifying them can help in understanding trends, detecting anomalies, or even in machine learning applications.
In this tutorial, we’ll explore various peak-finding algorithms using Python, providing you with practical examples and clear explanations. Whether you’re a beginner or an experienced programmer, this guide will enhance your skill set and give you the tools to tackle peak detection effectively. So, let’s dive into the world of peaks in Python and uncover how to implement these algorithms seamlessly.
Method 1: Using NumPy for Peak Detection
NumPy is a powerful library in Python that provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. One way to find peaks is by using the numpy
library, which can efficiently handle numerical data.
import numpy as np
import matplotlib.pyplot as plt
data = np.array([1, 3, 7, 1, 2, 6, 0, 5, 0, 4])
peaks = (data[1:-1] > data[:-2]) & (data[1:-1] > data[2:])
peak_indices = np.where(peaks)[0] + 1
plt.plot(data)
plt.plot(peak_indices, data[peak_indices], "x")
plt.title("Peak Detection using NumPy")
plt.xlabel("Index")
plt.ylabel("Value")
plt.show()
In this method, we first create a NumPy array containing our data points. We then identify peaks by checking if a point is greater than its neighbors. The boolean array peaks
captures this condition. Using np.where
, we find the indices of these peaks and adjust them to match the original data array. Finally, we visualize the data using Matplotlib, marking the detected peaks with ‘x’. This method is efficient and leverages NumPy’s capabilities for handling numerical computations.
Method 2: Using SciPy for Advanced Peak Detection
For more advanced peak detection, the scipy
library offers robust functionalities. The find_peaks
function from the scipy.signal
module is particularly useful for this purpose. It allows for more control over the peak detection process, including setting thresholds and minimum distance between peaks.
from scipy.signal import find_peaks
data = np.array([1, 3, 7, 1, 2, 6, 0, 5, 0, 4])
peaks, _ = find_peaks(data, height=3, distance=1)
plt.plot(data)
plt.plot(peaks, data[peaks], "x")
plt.title("Peak Detection using SciPy")
plt.xlabel("Index")
plt.ylabel("Value")
plt.show()
In this example, we utilize the find_peaks
function, which automatically identifies peaks based on the specified parameters. The height
parameter ensures that we only consider peaks above a certain threshold, while the distance
parameter prevents the detection of closely spaced peaks. This method is particularly powerful for noisy data, as it allows for fine-tuning the peak detection criteria. The resulting plot clearly illustrates the identified peaks, making it easy to visualize the results.
Method 3: Custom Peak Detection Algorithm
While libraries like NumPy and SciPy provide excellent tools for peak detection, sometimes you may want to implement a custom algorithm tailored to your specific needs. Below is a simple example of a custom peak detection algorithm using basic Python constructs.
def custom_peak_detection(data):
peaks = []
for i in range(1, len(data) - 1):
if data[i] > data[i - 1] and data[i] > data[i + 1]:
peaks.append(i)
return peaks
data = [1, 3, 7, 1, 2, 6, 0, 5, 0, 4]
peaks = custom_peak_detection(data)
print("Detected peaks at indices:", peaks)
Output:
Detected peaks at indices: [2, 5, 7]
In this custom implementation, we loop through the data points and check if each point is greater than its neighboring points. If it is, we append its index to the peaks
list. This method is straightforward and can be modified to include additional conditions or criteria based on your specific requirements. While it may not be as efficient as using optimized libraries, it provides a solid foundation for understanding the underlying logic of peak detection.
Conclusion
In conclusion, finding peaks in Python can be accomplished through various methods, each offering unique advantages. Whether you choose to leverage libraries like NumPy and SciPy for their efficiency and robustness or implement a custom algorithm for tailored solutions, understanding these techniques will enhance your data analysis capabilities. Peak detection plays a vital role in many applications, and mastering these methods will empower you to extract valuable insights from your data. So go ahead, experiment with these techniques, and elevate your Python programming skills!
FAQ
-
What is peak detection in Python?
Peak detection refers to the process of identifying local maxima in a dataset, which can help in analyzing trends and patterns. -
Which libraries are best for peak detection in Python?
NumPy and SciPy are two of the most popular libraries for peak detection due to their efficiency and extensive functionalities. -
Can I implement my own peak detection algorithm?
Yes, you can create custom peak detection algorithms using basic Python constructs, which can be tailored to your specific needs. -
How does the
find_peaks
function work in SciPy?
Thefind_peaks
function identifies peaks in a dataset based on specified parameters like height and distance, making it robust for various data types. -
Is peak detection useful in machine learning?
Yes, peak detection can help in feature extraction and anomaly detection, making it a valuable technique in machine learning applications.