How to Find Median of a List in Python
-
Use the
statistics
Module to Find the Median of a List in Python -
Use the
numpy.percentile
Function to Find the Median of a List in Python - Use the Custom Code to Find the Median of a List in Python
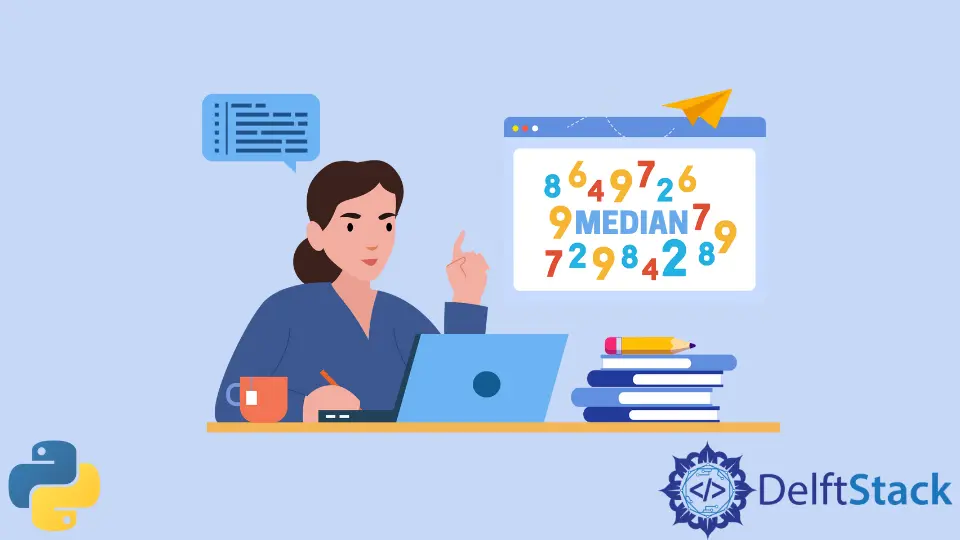
In the world of statistics and probability, the median value of a given set of observations is the middle element. It is calculated differently when the total number of elements is odd and even.
Python is frequently used for data and statistical analysis. This tutorial will introduce how to calculate the median of a list in Python.
Use the statistics
Module to Find the Median of a List in Python
In Python, we have the statistics
module with different functions and classes to find different statistical values from a set of data. The median()
function from this library can be used to find the median of a list.
Since the median is founded on a sorted list of data, the median()
function automatically sorts it and returns the median. For example,
import statistics
lst = [7, 8, 9, 5, 1, 2, 2, 3, 4, 5]
print(statistics.median(lst))
Output:
4.5
Use the numpy.percentile
Function to Find the Median of a List in Python
In the NumPy
module, we have functions that can find the percentile value from an array. The median of data is the 50th percentile value. To find this, we can use the percentile()
function from the NumPy
module and calculate the 50th percentile value. See the following code.
import numpy as np
a = np.array([7, 8, 9, 5, 1, 2, 2, 3, 4, 5])
median_value = np.percentile(a, 50)
print(median_value)
Output:
4.5
Use the Custom Code to Find the Median of a List in Python
We can also apply the formula to find the median of data using Python and create our user-defined function. For example,
lst = [7, 8, 9, 5, 1, 2, 2, 3, 4, 5]
def median(l):
half = len(l) // 2
l.sort()
if not len(l) % 2:
return (l[half - 1] + l[half]) / 2.0
return l[half]
print(median(lst))
Output:
4.5
The median of a list is the middle element of the sorted list if the list’s length is odd; otherwise, it is the average of the two middle elements.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn