How to Find Maximum and Minimum Value Using Lambda Expression in Python
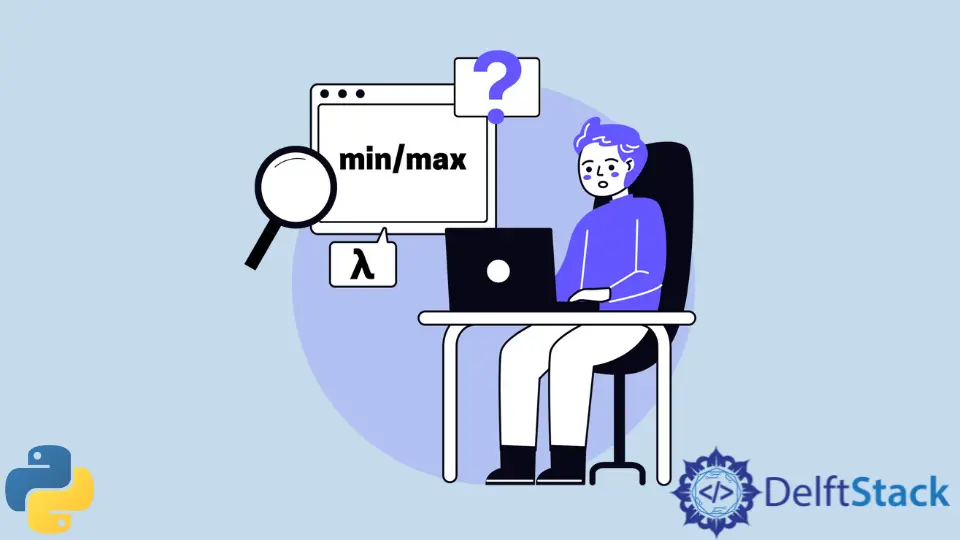
When we have a list of the wide range of values and want to find a maximum number in it in the most efficient way? We will use the lambda expression function of Python to find the maximum number for us rapidly. This article will introduce how to use the lambda expression to find the maximum and minimum number in the list using the key object in a lambda expression.
This lambda expression can be applied to the integer and strings by changing the key
object parameter of the lambda expression. In Python, the key
parameter compares the candidates of the list based on specific rules that are pre-defined in Python. Lambda expression can also be applied to the set of tuples used to store the ordered sequence of values efficiently.
There are various key functions in Python that return the desired values used for the sorting or ordering in the program. Some of the methods which accept the key as the object to manage how the program elements are ordered and grouped are: max()
, min()
, sort()
, and sorted()
, etc.
Find Maximum Value Using Lambda Expression
This technique will find the maximum number in the list using the lambda expression with the key
parameter. We will first initialize the values list with some random values in it (You can add any number in this list according to your requirements). Then we will call the max built-in function in Python and pass the key
parameter lambda and the value of type integer, it will return the maximum value from the list and then print it in the output console (You can then use this value to apply any logic).
Example Codes:
# python 3.x
valuesList = [222, 333, 444, 555, 2, 1]
print(max(valuesList, key=lambda value: int(value)))
Output:
555
Find Minimum Value Using Lambda Expression
This technique will find the minimum number in the list using the lambda expression with the key object. We will first initialize the values list with some random values (You can add any number in this list according to your requirements). Then we will call the min built-in function in Python and pass the key
parameter lambda and the value of type integer; it will return the minimum value from the list and then print it in the output console (You can then use this value to apply any logic).
Example Codes:
# python 3.x
valuesList = [222, 333, 444, 555, 2, 1]
print(min(valuesList, key=lambda value: int(value)))
Output:
1
Abdul is a software engineer with an architect background and a passion for full-stack web development with eight years of professional experience in analysis, design, development, implementation, performance tuning, and implementation of business applications.
LinkedIn