How to Find String in List in Python
- Method 1: Using List Comprehension
- Method 2: Using the filter() Function
- Method 3: Using Regular Expressions
- Conclusion
- FAQ
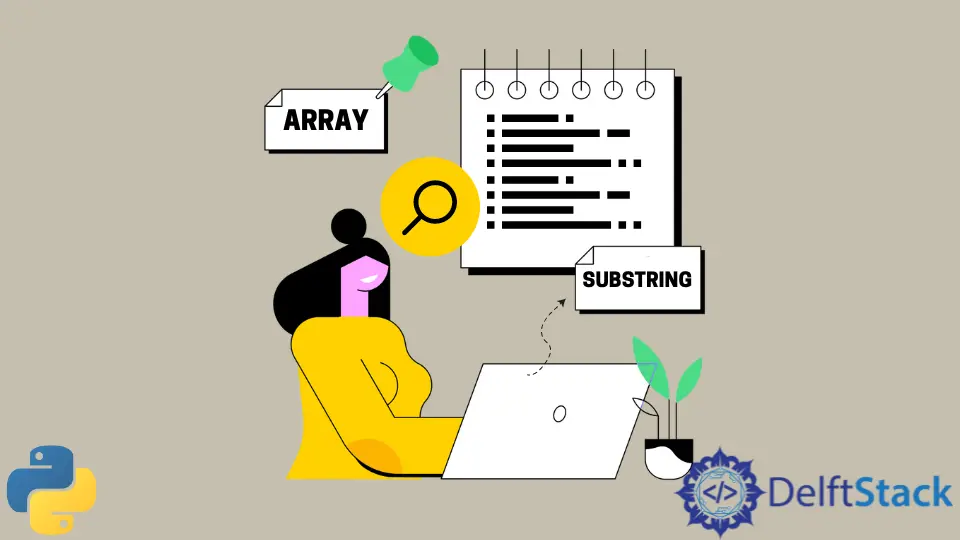
Finding elements within a list that contain a specific substring is a common task in Python programming. Whether you’re filtering data, searching for specific entries, or simply trying to locate items that match certain criteria, knowing how to effectively search through lists can significantly enhance your coding efficiency.
In this tutorial, we will explore various methods to find strings in lists using Python, providing clear examples and explanations to help you grasp each technique. By the end of this article, you’ll be equipped with the skills needed to handle string searches in your Python projects with ease.
Method 1: Using List Comprehension
List comprehension is a concise and efficient way to create lists in Python. It allows you to filter items based on a condition, making it perfect for finding strings that contain a specific substring.
Here’s how you can use list comprehension to find strings in a list:
my_list = ['apple', 'banana', 'cherry', 'date', 'fig', 'grape']
substring = 'ap'
found_items = [item for item in my_list if substring in item]
print(found_items)
Output:
['apple', 'grape']
In this example, we start with a list of fruit names. We define a substring, ‘ap’, and then use list comprehension to create a new list containing only those items that include the substring. The expression substring in item
checks each item in my_list
to see if it contains ‘ap’. The result is a new list, found_items
, which includes ‘apple’ and ‘grape’. This method is not only straightforward but also quite efficient, allowing you to quickly filter through large lists.
Method 2: Using the filter() Function
Another effective way to find strings in a list is by using the built-in filter()
function. This function constructs an iterator from elements of an iterable for which a function returns true. It can be combined with a lambda function to achieve the desired result.
Here’s how to use filter()
to find strings in a list:
my_list = ['apple', 'banana', 'cherry', 'date', 'fig', 'grape']
substring = 'an'
found_items = list(filter(lambda item: substring in item, my_list))
print(found_items)
Output:
['banana']
In this case, we again start with a list of fruit names. We define a substring, ‘an’, and use the filter()
function along with a lambda function to check each item. The lambda function returns True
if the substring is found in the item, thus filtering out the rest. The result is converted back to a list. The output shows ‘banana’, which is the only item containing ‘an’. This method is particularly useful when you want to apply more complex conditions or when working with larger datasets.
Method 3: Using Regular Expressions
For more complex string matching scenarios, Python’s re
module provides powerful tools through regular expressions. This method can be particularly useful if you need to find patterns or perform case-insensitive searches.
Here’s how to find strings using regular expressions:
import re
my_list = ['apple', 'banana', 'cherry', 'date', 'fig', 'grape']
substring = 'a'
found_items = [item for item in my_list if re.search(substring, item)]
print(found_items)
Output:
['apple', 'banana', 'grape']
In this example, we import the re
module and define our list of fruit names. The substring ‘a’ is our target. Using list comprehension, we apply re.search()
to check if the substring exists within each item. The re.search()
function returns a match object if the substring is found, making it suitable for more complex searches. The output includes ‘apple’, ‘banana’, and ‘grape’, demonstrating the flexibility of regular expressions for string searching in lists.
Conclusion
In this tutorial, we explored three effective methods for finding strings in lists using Python: list comprehension, the filter()
function, and regular expressions. Each approach has its own advantages, depending on your specific needs and the complexity of the search criteria. By mastering these techniques, you can streamline your data processing tasks and enhance your programming skills. Whether you’re working on small scripts or larger applications, knowing how to efficiently search through lists will undoubtedly be a valuable asset in your toolkit.
FAQ
- What is list comprehension in Python?
List comprehension is a concise way to create lists based on existing lists by applying a condition.
-
How does the filter() function work?
The filter() function creates an iterator from elements of an iterable for which a function returns true. -
When should I use regular expressions?
Regular expressions are useful for complex string matching and pattern searching. -
Can I perform case-insensitive searches?
Yes, you can use the re.IGNORECASE flag with regular expressions for case-insensitive searches. -
Are there any performance differences between these methods?
Yes, performance can vary based on the size of the list and complexity of the conditions, so testing is advisable.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python