How to Find Element by Text Using Selenium in Python
- Method 1: Using XPath to Find Element by Text
- Method 2: Using CSS Selectors to Find Element by Text
- Method 3: Using Link Text and Partial Link Text
- Conclusion
- FAQ
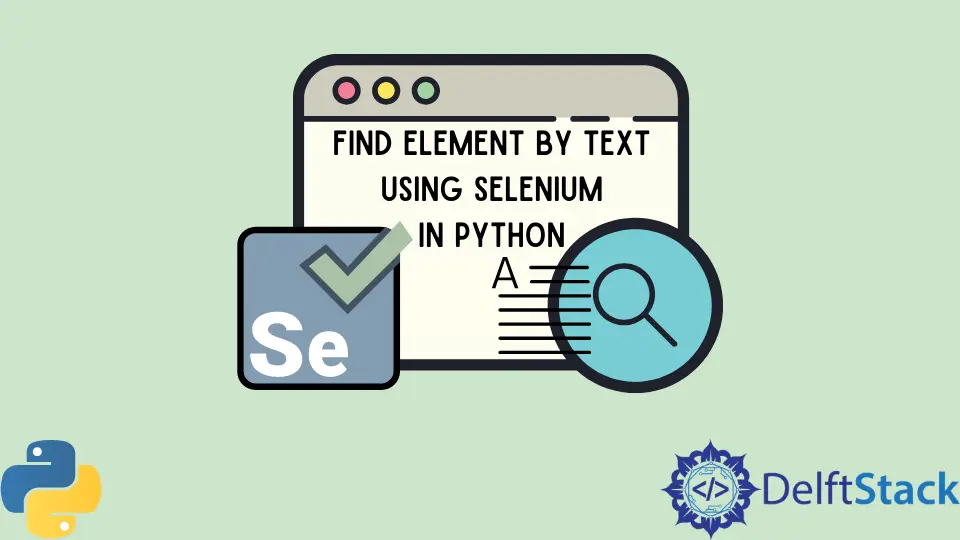
When it comes to web automation and testing, Selenium is a powerful tool that allows developers to interact with web pages programmatically. One common requirement is finding elements on a page by their visible text. This can be particularly useful when the HTML structure is complex, and you want to ensure you’re targeting the right element.
In this tutorial, we will explore various methods to find elements by text using Selenium in Python. Whether you’re a beginner or an experienced developer, this guide will provide you with the knowledge you need to enhance your web automation skills.
Method 1: Using XPath to Find Element by Text
XPath is a powerful language for navigating through elements and attributes in an XML document. In Selenium, it can be used to locate elements on a web page based on their text content. The syntax for finding an element by text using XPath is straightforward. Here’s how you can do it:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('http://example.com')
element = driver.find_element_by_xpath("//*[text()='Your Text Here']")
print(element.text)
driver.quit()
In this code snippet, we first import the necessary Selenium module and create a new instance of the Chrome driver. After navigating to the desired URL, we use the find_element_by_xpath
method to locate the element containing the specified text. The XPath expression //*[text()='Your Text Here']
searches for any element (*
) that has the exact text specified. Once the element is found, we print its text to confirm that we have the correct element. Finally, we close the browser using driver.quit()
.
Output:
Your Text Here
Using XPath is advantageous because it allows you to specify not only the text but also conditions for the element’s attributes or position in the DOM, making it a versatile option for more complex queries.
Method 2: Using CSS Selectors to Find Element by Text
While XPath is powerful, CSS selectors can also be used to find elements by text, though it requires a slightly different approach. CSS does not support direct text matching, so we need to combine it with JavaScript execution. Here’s how you can achieve this:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('http://example.com')
element = driver.execute_script("return [...document.querySelectorAll('*')].find(el => el.textContent === 'Your Text Here');")
print(element.text)
driver.quit()
In this example, we again start by importing the Selenium module and creating a new Chrome driver instance. After navigating to the desired web page, we execute a JavaScript snippet that selects all elements and filters them to find the one with the specified text. The textContent
property is used to compare the text of each element. Once found, we print the text of the element to verify our selection. Finally, we close the browser.
Output:
Your Text Here
This method combines the power of CSS selectors with JavaScript, allowing for a more flexible approach when dealing with text-based element searches. It can be particularly useful in scenarios where you need to filter elements based on dynamic content.
Method 3: Using Link Text and Partial Link Text
If you’re specifically looking for links, Selenium provides convenient methods to find elements by their link text or partial link text. This is particularly useful for navigating websites with many hyperlinks. Here’s how you can use these methods:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('http://example.com')
element = driver.find_element_by_link_text('Full Link Text')
print(element.text)
# For partial link text
partial_element = driver.find_element_by_partial_link_text('Partial Text')
print(partial_element.text)
driver.quit()
In this code, we navigate to a webpage and use find_element_by_link_text
to locate a link with the exact text. If you only know part of the link text, find_element_by_partial_link_text
allows you to find the link based on a substring. After locating the elements, we print their text to confirm our selection. Finally, we close the browser.
Output:
Full Link Text
Partial Text
Using link text methods is straightforward and efficient, especially when dealing with navigation menus or any area with multiple hyperlinks. These methods are specifically tailored for link elements, making them a great choice when your target element is a hyperlink.
Conclusion
Finding elements by their text using Selenium in Python can significantly enhance your web automation capabilities. Whether you choose to use XPath, CSS selectors, or link text methods, each approach has its strengths and can be applied based on the specific needs of your project. By mastering these techniques, you will be able to navigate complex web pages more effectively and create robust automated tests. Happy coding!
FAQ
-
How do I install Selenium for Python?
You can install Selenium using pip by running the command pip install selenium in your terminal or command prompt. -
What browsers are supported by Selenium?
Selenium supports various browsers, including Chrome, Firefox, Safari, and Edge, among others. -
Can I use Selenium with headless browsers?
Yes, Selenium can be configured to run in headless mode, allowing you to automate browser tasks without a visible UI. -
What is the difference between find_element_by_xpath and find_element_by_css_selector?
find_element_by_xpath uses XPath syntax to locate elements, while find_element_by_css_selector uses CSS selector syntax. Both can be used to find elements, but XPath is more powerful for complex queries. -
Is Selenium suitable for testing single-page applications (SPAs)?
Yes, Selenium can be used for testing SPAs, but you may need to implement additional waits and checks to handle dynamic content loading.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python Selenium
- How to Check if Element Exists Using Selenium Python
- How to Refresh Page in Python Selenium
- WebDriverException: Message: Geckodriver Executable Needs to Be in PATH Error in Python
- How to Install Python Selenium in macOS
- How to Login to a Website Using Selenium Python
- How to Open and Close Tabs in a Browser Using Selenium Python