How to Exit From the Python Command Line
Vaibhav Vaibhav
Feb 02, 2024
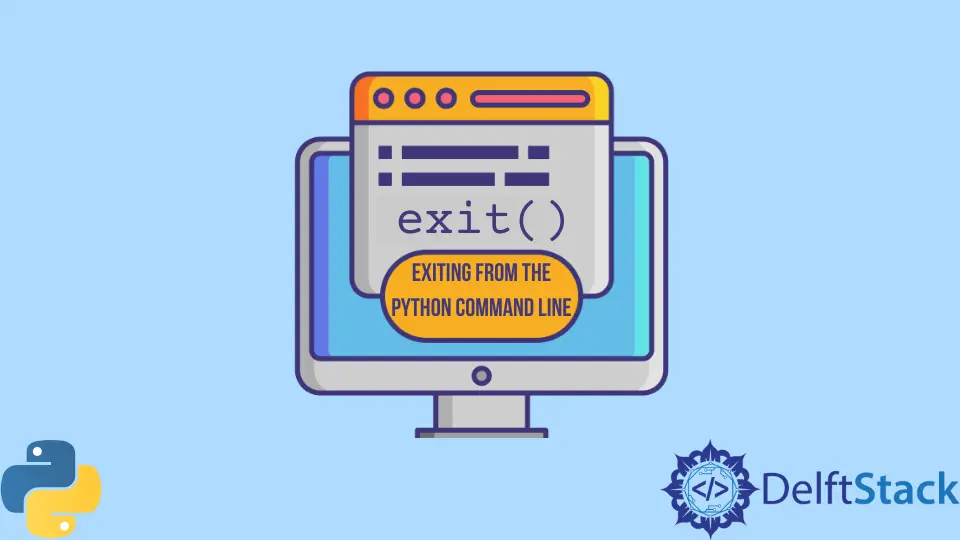
Python Interpreter can be activated from any command line by typing python
. Once activated, it can be used to write code and run it. Once the interpreter has started, the usual command-line utilities can’t be used unless we exit from the Python interpreter. There are a few ways to exit the Python interpreter. This article talks about them.
Ways to Exit the Python Interpreter
Following are the ways to exit the Python Interpreter.
- Type
exit()
: Note that it isexit()
but notexit
. It is a function call. - On Windows, use the key combo Ctrl + Z + Enter.
- On UNIX Systems, use the key combo Ctrl + D.
Author: Vaibhav Vaibhav