How to Exit Codes in Python
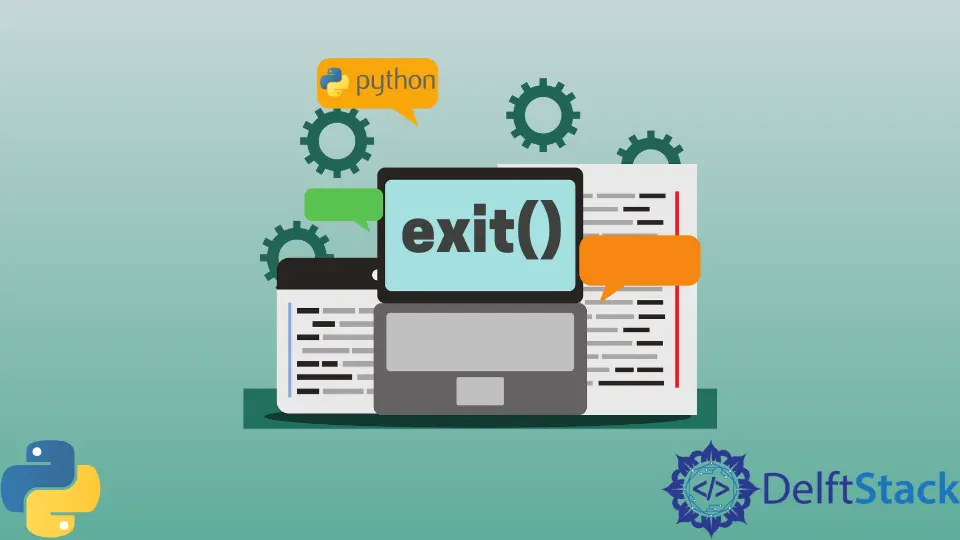
If you’re a programmer or a developer, you must have come across the term exit code. Think of exit code as messages which the programs usually send to the operating system, and in some cases, to other programs.
Exit codes tell the operating system or other programs about its success or failure. When no error occurs in the execution of the program, it is known as success. And, when some error occurs, it is known as a failure.
Different kind of situations has different exit codes. Generally, we only use two exit codes, namely, 0 and 1. 0
refers to successful execution, and 1
refers to a failed execution.
If you’ve ever worked with C or C++, you’d know that the main()
function is always ended with a return 0;
statement. This statement says that if the program executes error-free, then return a positive or a success status or message to the operating system.
Exit Codes in Python Using sys
Even though we don’t mention these exit codes explicitly in the code in Python, that doesn’t mean that Python doesn’t have these exit codes. All the programming languages have exit codes.
We use the built-in sys
module to implement exit codes in Python.
The sys
module has a function, exit()
, which lets us use the exit codes and terminate the programs based on our needs. The exit()
function accepts a single argument which is the exit code itself. The default value of the argument is 0
, that is, a successful response.
For example, refer to the following program.
import sys
print("Hello World")
sys.exit(0)
print("Hello World 2.0")
Output:
Hello World
The program above just prints Hello World
because the sys.exit(0)
terminates the program before the interpreter could even reach the last print statement. So, you can use this statement where ever you wish to end the program abruptly.