How to Examine Items in a Python Queue
- Understanding the Queue Module
- Examining Items with the Queue Class
- Using the Deque Class for More Flexibility
- Peeking at the First Item in the Queue
- Conclusion
- FAQ
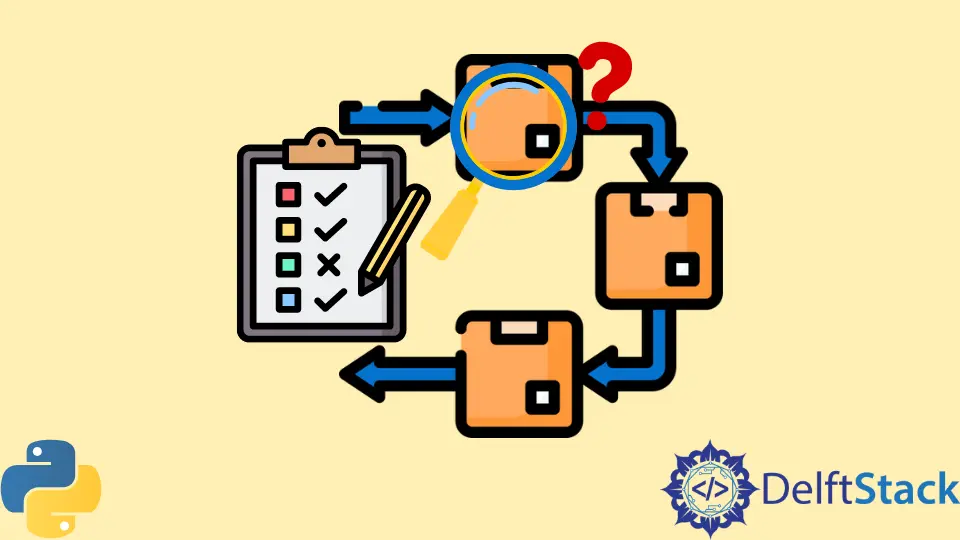
In the realm of programming, managing data efficiently is key to building robust applications. One of the fundamental structures used for this purpose is the queue. In Python, the queue
module provides a simple way to implement queues and deques, enabling you to handle data in a first-in, first-out (FIFO) manner.
This tutorial will guide you through the process of examining items in a Python queue, showcasing how to utilize the Queue
and deque
functions effectively. Whether you’re managing tasks in a multi-threaded environment or simply organizing data, understanding how to manipulate queues can vastly improve your programming skills. Let’s dive into the methods to examine items within a queue in Python.
Understanding the Queue Module
Before we jump into the methods, it’s essential to understand what a queue is and how the queue
module works. A queue is a collection that follows the FIFO principle, meaning that the first item added to the queue will be the first one to be removed. Python’s queue
module provides a thread-safe implementation of queues, making it particularly useful in multi-threaded applications.
To use the queue, you’ll need to import the Queue
class from the queue
module. Here’s a simple example of how to create and interact with a queue:
from queue import Queue
my_queue = Queue()
my_queue.put(1)
my_queue.put(2)
my_queue.put(3)
print(my_queue.queue)
Output:
deque([1, 2, 3])
In this code, we first import the Queue
class. We then create a queue called my_queue
and add three items to it. Finally, we print the content of the queue, which shows the items in the order they were added.
Examining Items with the Queue Class
To examine items in a queue, the Queue
class provides several methods. One of the most straightforward ways to peek at the items without removing them is by accessing the internal queue directly. While this method is not typically recommended for production code due to encapsulation concerns, it can be useful for debugging or simple applications.
Here’s how you can examine items in a queue using the queue
attribute:
from queue import Queue
my_queue = Queue()
my_queue.put(1)
my_queue.put(2)
my_queue.put(3)
items = list(my_queue.queue)
print(items)
Output:
[1, 2, 3]
This example demonstrates how to convert the internal queue into a list, allowing you to see all the items currently in the queue. By accessing my_queue.queue
, we retrieve the underlying deque. Converting it to a list gives us a clear view of the items without modifying the queue itself.
Using the Deque Class for More Flexibility
If you require more flexibility than what the Queue
class offers, you might want to consider using the deque
class from the collections
module. Deques are double-ended queues, allowing you to add and remove items from both ends efficiently.
Here’s how to create and examine items in a deque:
from collections import deque
my_deque = deque()
my_deque.append(1)
my_deque.append(2)
my_deque.append(3)
print(my_deque)
Output:
deque([1, 2, 3])
In this code snippet, we create a deque called my_deque
and add three items to it using the append
method. When we print my_deque
, it shows the items in the order they were added. The deque
class provides additional methods like appendleft
, pop
, and popleft
, offering greater versatility in managing your data.
Peeking at the First Item in the Queue
Sometimes, you may want to access the first item in a queue without removing it. While the Queue
class doesn’t provide a direct method for peeking, we can achieve this by accessing the first element of the internal queue. Here’s how to do it:
from queue import Queue
my_queue = Queue()
my_queue.put(1)
my_queue.put(2)
my_queue.put(3)
first_item = my_queue.queue[0]
print(first_item)
Output:
1
In this example, we simply access the first item in the queue by indexing into the internal deque
. This approach allows you to peek at the first item without altering the queue’s state, making it a useful technique when you need to check what’s next in line.
Conclusion
Examining items in a Python queue is a fundamental skill that can enhance your programming toolkit. Whether you choose to use the Queue
class for thread-safe operations or the more flexible deque
from the collections
module, understanding how to manage and inspect your data structures is crucial. By implementing the methods discussed in this tutorial, you’ll be better equipped to handle various programming challenges involving queues. Keep practicing, and soon you’ll find yourself navigating through queues with ease.
FAQ
-
What is the difference between Queue and deque in Python?
Queue is a thread-safe implementation for FIFO operations, while deque allows for faster appends and pops from both ends. -
Can I access items in a Queue without removing them?
Yes, you can access items by using the internal queue attribute, but this is generally not recommended for production code. -
How do I remove an item from a queue?
Use theget()
method to remove and return an item from the front of the queue. -
Is deque thread-safe?
No, the deque class is not thread-safe, which means you should handle synchronization if used in multi-threaded scenarios.
- Can I use a queue for multi-threading?
Yes, the Queue class is designed for multi-threading and provides methods that are safe to use across different threads.
Abdul is a software engineer with an architect background and a passion for full-stack web development with eight years of professional experience in analysis, design, development, implementation, performance tuning, and implementation of business applications.
LinkedIn