The eval() Function in Python
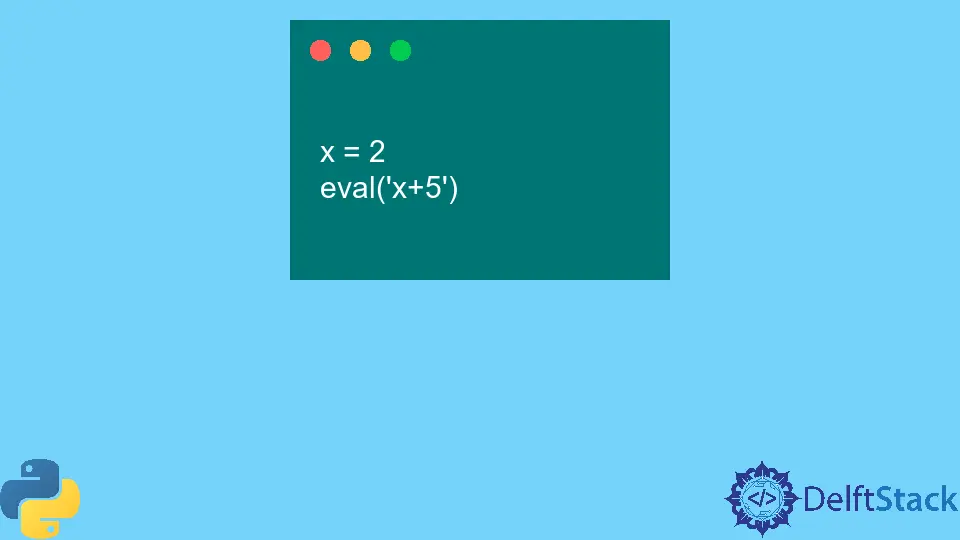
Python is an interpreted high-level programming language. It has a clean code structure and readability with its noticeable significant indentation.
The eval()
function parses the expression argument and then evaluates it as a python expression.
Syntax of the eval()
function is provided below.
eval(expression, [globals[, locals]])
The return value will be based on and will be the result of the evaluated expression.
We evaluate a simple addition in the example below.
x = 2
eval("x+5")
Output:
7
The eval()
function arguments are a string and optional arguments of globals
and locals
. If globals
are provided, it must be a dictionary. The locals
can be any mapping object.
Suppose the globals
dictionary is provided, and the value for the key is not provided with that. A reference is generated to the dictionary of the built-in module builtins
, and inserted under the key section before the expression starts parsing.
This roughly suggests that the expression has typically complete access to the standard builtins
module. If the locals
object is not provided, it defaults down to the global dictionary. Also, in a scenario where both the dictionaries are omitted, the expression does execute with the globals
and locals
in the environment where the function eval()
is called.
There are certain functions present inside the builtins
module which can probably damage the system significantly. But it is certainly possible to block any of the stuff that may be suspicious.
For example, consider an example to create a list representing the number of available cores inside the system.
from os import cpu_count
eval("[1, cpu_count()]")
Output:
[1, 8]
eval(input())
will ask the user to enter the string and execute it as code. If the os
module is imported, one can perform all kinds of actions on the device, like deleting files and tampering with the system.