The which Command in Python
-
Use the
shutil.which()
Function to Emulate thewhich
Command in Python -
Create a Function to Emulate the
which
Command in Python
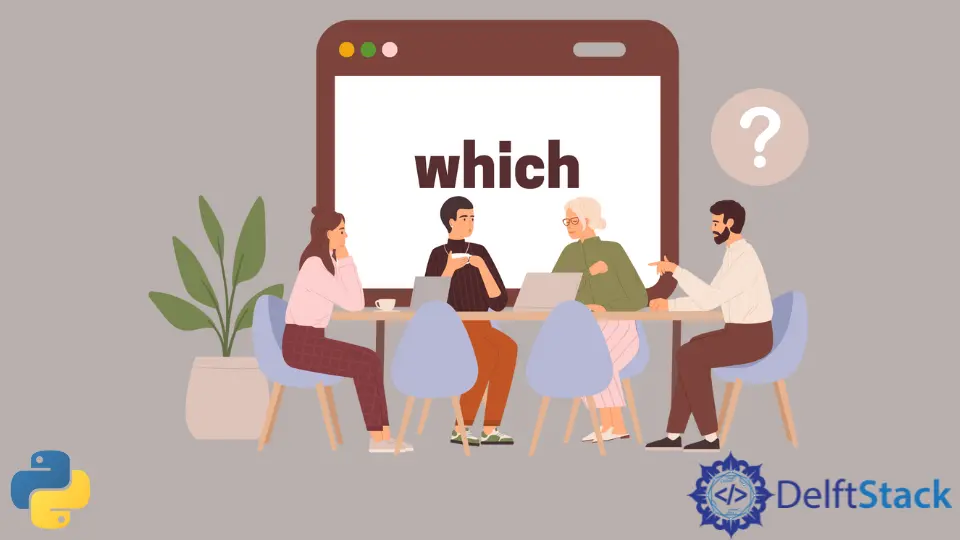
In Linux, we have the which
command. This command can identify the path for a given executable.
In this tutorial, we will emulate this command in Python.
Use the shutil.which()
Function to Emulate the which
Command in Python
We can emulate this command in Python using the shutil.which()
function. This function is a recent addition in Python 3.3. The shutil
module offers several functions to deal with the operations on files and to their collections.
The shutil.which()
function returns the path of a given executable, which would run if cmd
was called.
For example,
import shutil
print(shutil.which("python"))
Output:
C:\Anaconda\python.EXE
In the above example, the shutil.which()
returns the directory of the Python executable.
Create a Function to Emulate the which
Command in Python
Below Python 3.3, there is no way to use the shutil.which()
function. So here, we can create a function using functions from the os
module (os.path.exists()
) and os.access
methods) to search for the given executable and emulate the which
command.
See the following code.
import os
def which(pgm):
path = os.getenv("PATH")
for p in path.split(os.path.pathsep):
p = os.path.join(p, pgm)
if os.path.exists(p) and os.access(p, os.X_OK):
return p
print(which("python.exe"))
Output:
C:\Anaconda\python.exe