How to dotenv in Python
- What is Python Dotenv?
- Creating a .env File
- Loading Environment Variables in Python
- Best Practices for Using Python Dotenv
- Conclusion
- FAQ
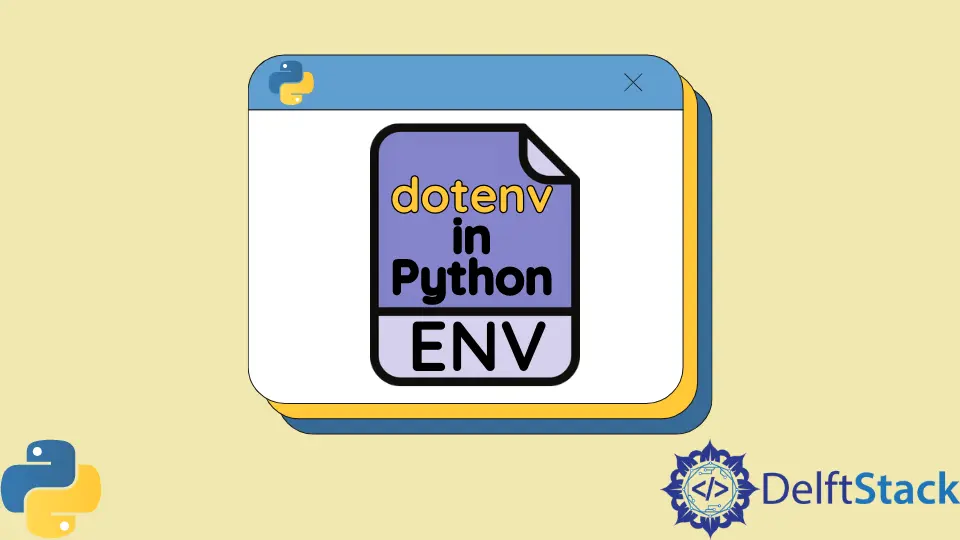
When developing applications in Python, managing environment variables is crucial for maintaining security and flexibility. This is where the Python Dotenv library comes into play. It allows you to load environment variables from a .env
file into your application, keeping sensitive information like API keys and database credentials secure and separate from your codebase.
In this tutorial, we will dive into how to use Python Dotenv effectively, exploring installation, usage, and best practices. Whether you’re a beginner or an experienced developer, this guide will equip you with the knowledge you need to implement dotenv in your Python projects seamlessly.
What is Python Dotenv?
Python Dotenv is a library that simplifies the management of environment variables in your applications. By creating a .env
file, you can store key-value pairs that represent your environment variables. This is particularly useful for applications that require different configurations for development, testing, and production environments. By using Python Dotenv, you can avoid hardcoding sensitive information directly into your code, which is a significant security risk.
To get started, you’ll need to install the Python Dotenv package. You can easily do this using pip, Python’s package installer.
pip install python-dotenv
Output:
Successfully installed python-dotenv
Once installed, you can begin using it in your Python scripts. The next step is to create a .env
file in your project directory, where you will define your environment variables.
Creating a .env File
Creating a .env
file is straightforward. Open your favorite text editor and create a new file named .env
in your project directory. Inside this file, you can define your environment variables in the format of KEY=VALUE
. For example:
DATABASE_URL=postgres://user:password@localhost/dbname
API_KEY=your_api_key_here
SECRET_KEY=your_secret_key_here
Once you’ve defined your environment variables, you can load them into your Python application using the dotenv
library.
Loading Environment Variables in Python
Now that you have your .env
file set up, the next step is to load these variables into your Python application. This is done using the load_dotenv
function from the dotenv
module. Here’s how you can do it:
from dotenv import load_dotenv
import os
load_dotenv()
database_url = os.getenv('DATABASE_URL')
api_key = os.getenv('API_KEY')
secret_key = os.getenv('SECRET_KEY')
print(database_url)
print(api_key)
print(secret_key)
Output:
postgres://user:password@localhost/dbname
your_api_key_here
your_secret_key_here
In this snippet, we first import the necessary modules. The load_dotenv()
function reads the .env
file and loads the variables into the environment. We then use os.getenv()
to access these variables, allowing us to use them throughout our application. This method keeps your sensitive information secure and easily manageable.
Best Practices for Using Python Dotenv
While using Python Dotenv is straightforward, there are some best practices you should follow to ensure your application remains secure and maintainable:
-
Never commit your .env file to version control: Since the
.env
file contains sensitive information, it’s crucial to add it to your.gitignore
file. This prevents it from being accidentally shared in public repositories. -
Use default values: When accessing environment variables, it’s a good idea to provide default values. This ensures your application can still run even if certain environment variables are not set.
-
Document your environment variables: Keeping a separate documentation file that outlines what each environment variable does can be extremely helpful for other developers or for future reference.
-
Consider using a secrets manager: For production environments, consider using a dedicated secrets management tool instead of relying solely on a
.env
file. Tools like AWS Secrets Manager or HashiCorp Vault provide enhanced security features.
By following these best practices, you can ensure that your application remains both secure and easy to manage.
Conclusion
In summary, using Python Dotenv is an effective way to manage environment variables in your applications. By loading sensitive information from a .env
file, you can enhance security and maintainability. With the steps outlined in this guide, you’re now equipped to implement dotenv in your Python projects confidently. Remember to follow best practices to keep your application secure and efficient. Happy coding!
FAQ
-
What is the purpose of the .env file in Python Dotenv?
The .env file is used to store environment variables in key-value pairs, allowing you to manage sensitive information securely. -
How do I install Python Dotenv?
You can install Python Dotenv using pip with the command pip install python-dotenv. -
Can I use Python Dotenv in production?
Yes, Python Dotenv can be used in production, but consider using a dedicated secrets management tool for enhanced security.
-
Is it safe to store API keys in a .env file?
While storing API keys in a .env file is safer than hardcoding them in your code, ensure that the .env file is not committed to version control. -
How can I access environment variables in Python?
You can access environment variables in Python using the os.getenv() function after loading the .env file with load_dotenv().