Difference Between typing.Dict & Dict and Their Uses in Python
- Understanding dict
- Exploring typing.Dict
- Comparison of dict and typing.Dict
- Practical Uses of dict and typing.Dict
- Conclusion
- FAQ
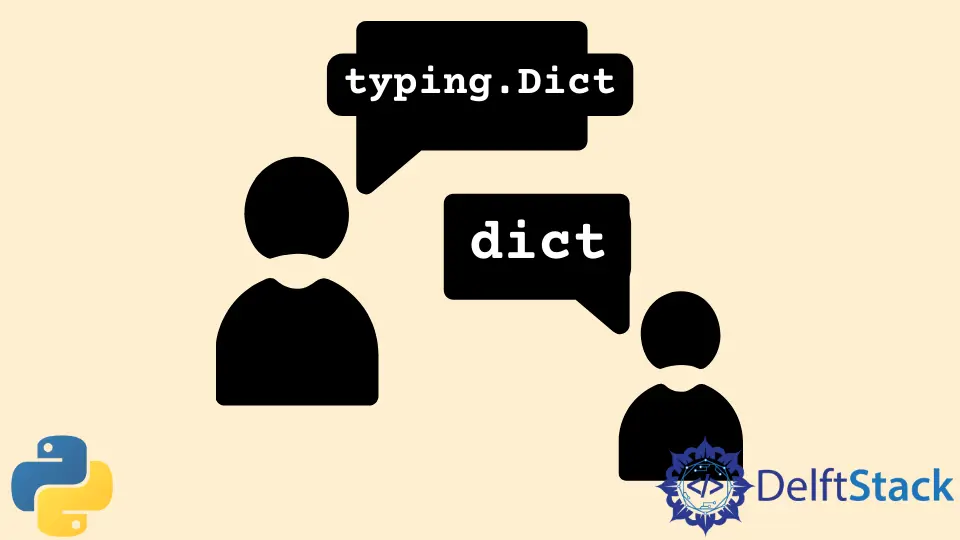
In the ever-evolving world of Python programming, understanding the nuances of data types is crucial for writing efficient and maintainable code. One such nuance is the distinction between typing.Dict
and the built-in dict
. Both serve the fundamental purpose of storing key-value pairs, but they cater to different needs and scenarios in type hinting and static type checking.
This article delves into the differences between typing.Dict
and dict
, illustrating their unique uses and providing examples to clarify their applications. Whether you’re a novice looking to enhance your coding skills or an experienced developer aiming to refine your type annotations, this guide will equip you with the knowledge needed to leverage these powerful tools effectively.
Understanding dict
The built-in dict
in Python is a versatile and widely-used data structure that allows for the storage of key-value pairs. It is mutable, meaning that you can change its content without creating a new object. dict
is a core component of Python, making it essential for many applications, from simple data storage to complex data manipulation.
Here’s a basic example of how to create and use a dict
in Python:
my_dict = {
"name": "Alice",
"age": 30,
"city": "New York"
}
print(my_dict)
Output:
{'name': 'Alice', 'age': 30, 'city': 'New York'}
In this example, my_dict
stores three key-value pairs: a name, an age, and a city. You can access values using their corresponding keys, modify them, or even add new pairs. The flexibility of dict
makes it an ideal choice for dynamic data storage, especially when the structure of the data is not fixed.
The built-in dict
does not enforce any type restrictions on its keys or values, which means you can mix different data types. However, this lack of enforcement can lead to potential errors if you expect certain types but receive others. This is where typing.Dict
comes into play.
Exploring typing.Dict
typing.Dict
is part of Python’s type hinting system introduced in PEP 484. It provides a way to specify the types of keys and values that a dictionary can hold, enhancing code readability and helping with static type checking. Using typing.Dict
helps developers understand the expected data structure at a glance, making the code more maintainable.
Here’s an example of how to declare a typing.Dict
:
from typing import Dict
my_typed_dict: Dict[str, int] = {
"Alice": 30,
"Bob": 25,
}
print(my_typed_dict)
Output:
{'Alice': 30, 'Bob': 25}
In this case, my_typed_dict
is explicitly defined to have string keys and integer values. This type hinting helps tools like mypy perform static type checks, catching potential errors before runtime. If you try to add a non-integer value, such as a string, mypy will raise an error, thus enforcing type safety.
The use of typing.Dict
is particularly beneficial in larger codebases where the complexity of data structures can lead to confusion. By providing clear type hints, you can ensure that your code is both robust and understandable to others (or even to yourself in the future).
Comparison of dict and typing.Dict
While both dict
and typing.Dict
serve the purpose of storing key-value pairs, they differ significantly in their approach to type safety and clarity. The built-in dict
is straightforward and flexible, allowing for any data type as keys or values. However, this flexibility can lead to runtime errors if the data types do not match expected values.
On the other hand, typing.Dict
promotes a more structured approach by enforcing type hints. This can prevent bugs and improve code quality, especially in collaborative environments where multiple developers may be working on the same codebase. By using typing.Dict
, you can communicate your intentions more clearly, making it easier for others to understand how to interact with your data structures.
To summarize, here are the key differences:
dict
is versatile and flexible, whiletyping.Dict
enforces type hints for better clarity and safety.dict
allows any data type as keys and values, whereastyping.Dict
requires specified types.typing.Dict
aids in static type checking, reducing potential runtime errors.
Practical Uses of dict and typing.Dict
Understanding when to use dict
versus typing.Dict
can significantly impact your Python programming. The built-in dict
is perfect for scenarios where the structure of your data is dynamic or not strictly defined. For example, if you are working with JSON data or user-generated content where the keys and values may vary, using dict
allows for greater flexibility.
Here’s a quick example demonstrating dynamic data handling with dict
:
user_data = {}
user_data['user1'] = {'name': 'Alice', 'age': 30}
user_data['user2'] = {'name': 'Bob', 'age': 25}
print(user_data)
Output:
{'user1': {'name': 'Alice', 'age': 30}, 'user2': {'name': 'Bob', 'age': 25}}
In this case, user_data
is a dictionary that can hold various user profiles without any pre-defined structure. This is particularly useful in applications like web development, where user data can be unpredictable.
Conversely, typing.Dict
is ideal for scenarios where data types are known and should be enforced. This is particularly useful in APIs, data processing, and applications where data integrity is paramount.
Consider the following example where you want to ensure that a function receives a dictionary with specific types:
from typing import Dict
def get_user_age(users: Dict[str, int]) -> int:
return users.get("Alice", 0)
user_ages: Dict[str, int] = {"Alice": 30, "Bob": 25}
print(get_user_age(user_ages))
Output:
30
Here, the function get_user_age
expects a typing.Dict
with string keys and integer values. This ensures that any data passed to the function adheres to the expected structure, preventing potential errors.
Conclusion
In conclusion, both typing.Dict
and dict
have their unique places in Python programming. While dict
offers flexibility for dynamic data structures, typing.Dict
provides the structure and type safety needed for more complex applications. Understanding when to use each can enhance your coding practices, making your code more robust and easier to maintain. As you continue to develop your Python skills, consider leveraging these tools to improve your code quality and reduce potential errors.
FAQ
-
What is the main difference between dict and typing.Dict?
dict is a built-in data structure that allows for any type of keys and values, while typing.Dict enforces type hints for keys and values. -
When should I use typing.Dict?
Use typing.Dict when you want to enforce specific data types for keys and values, especially in larger codebases or when collaborating with others.
-
Can I mix data types in typing.Dict?
No, typing.Dict requires you to specify the types of keys and values, which helps prevent mixing incompatible data types. -
Is typing.Dict available in all Python versions?
typing.Dict is available in Python 3.5 and later versions as part of the typing module. -
How does using typing.Dict improve code quality?
By enforcing type hints, typing.Dict helps catch potential errors during static type checking, making your code more reliable and easier to understand.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn