How to Fix AttributeError: 'Dict' Object Has No Attribute 'Append' in Python
-
the
AttributeError: 'dict' object has no attribute 'append'
in Python -
Handle the
AttributeError: 'dict' object has no attribute 'append'
in Python
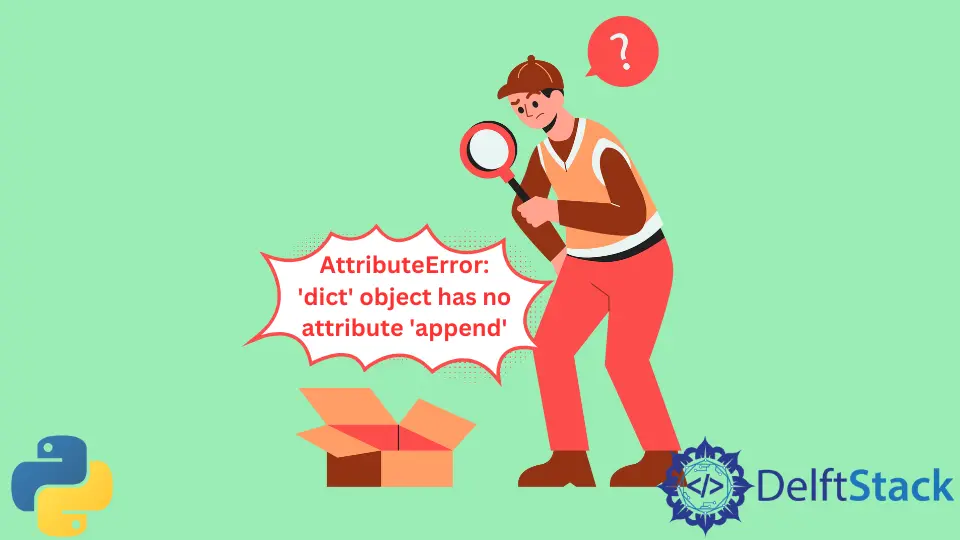
dict
is a data structure that uses the hash map, which differs from the list. It doesn’t have the append()
function, while the list data structure has the append()
function.
the AttributeError: 'dict' object has no attribute 'append'
in Python
Dictionary can hold a list inside it. We can’t directly append the dictionary, but if there’s a list inside the dictionary, we can easily append that.
For example,
>>> dict = {}
>>> dict["numbers"]=[1,2,3]
>>> dict["numbers"]
[1, 2, 3]
>>> dict["numbers"].append(4)
>>> dict["numbers"]
[1, 2, 3, 4]
Here, the numbers
key has a list as the value. We can append this, but let’s say we want to append the dict
.
It will show the following error:
>>> dict = {}
>>> dict["numbers"]=[1,2,3]
>>> dict.append(12)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'dict' object has no attribute 'append'
Handle the AttributeError: 'dict' object has no attribute 'append'
in Python
The value can be anything: a tuple, list, string, or even another dictionary. To prevent this error, we can check the type of value for a particular key inside a dictionary.
For this, we need to evaluate whether the key exists inside the dictionary or not.
Let’s see the example below:
dict = {}
dict["nums"] = [1, 2, 3]
dict["tuple"] = (1, 2, 3)
dict["name"] = "Alex"
if dict.get("name", False):
if type(dict["name"]) is list:
dict["name"].append("Another Name")
else:
print("The data type is not a list")
else:
print("This key isn't valid")
Output:
The data type is not a list
It could give us the error like the previous one, but we are evaluating the dictionary’s key here. Then we check if the value is a list or not.
After that, we are appending the list.
To know more about the Python dictionary, read this blog.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python