How to Convert Radians to Degrees and Vice-Versa in Python
-
Use the
math
Module in Python to Convert Degree to Radian and Vice-Versa -
Use the
NumPy
Module to Convert Degree to Radian and Vice-Versa
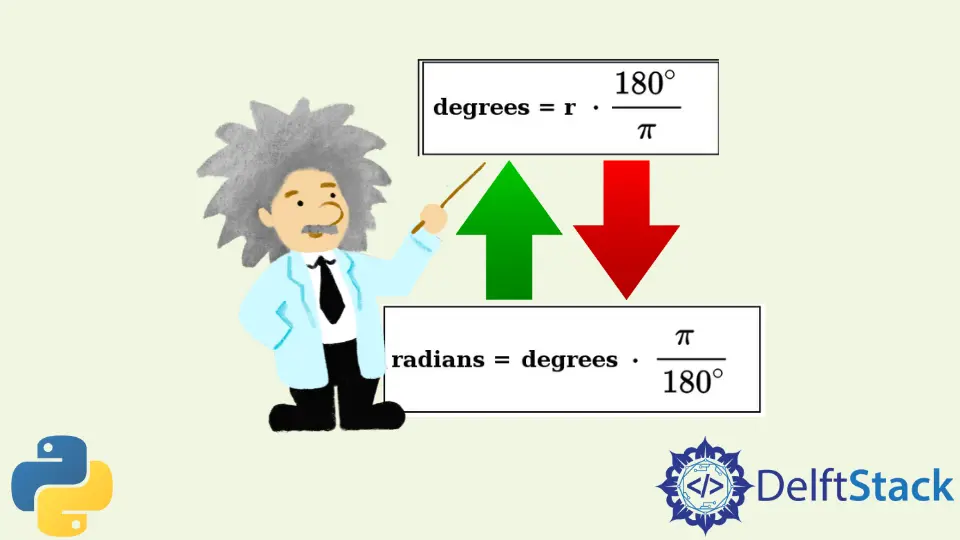
Degrees and radians are two of the highly used units to represent angles. The representation between these two units is shown below.
In this tutorial, we will discuss how to convert degree to radian and vice-versa.
Use the math
Module in Python to Convert Degree to Radian and Vice-Versa
It is straightforward to implement their relations manually in Python. We can use the math
library for the required constants if we are not familiar with them. For example,
print((math.pi / 2) * 180.0 / math.pi) # Rad to Deg
print(90 * math.pi / 180.0) # Deg to Rad
Output:
90.0
1.5707963267948966
Note that the math.pi
returns the mathematical constant pi and can be replaced by its value 3.141592….
We can also use different functions from the math
library to carry out these conversions.
The math.degrees()
function will convert the radian value passed to it into degree. For example,
import math
print(math.degrees(math.pi / 2))
Output:
90.0
The math.radians()
function will do the opposite and convert the degree value to radians. For example,
import math
print(math.radians(90))
Output:
1.5707963267948966
Use the NumPy
Module to Convert Degree to Radian and Vice-Versa
The NumPy
module is also equipped with different functions to convert between radian and degree values. The numpy.degrees()
function converts radians to degree and can accept an array or list of values at once.
Similarly, the numpy.radians()
function converts degree to radians and can also accept an array or list of values.
The following code shows an example of these two functions.
import numpy
lst1 = [math.pi / 2, math.pi]
print(numpy.degrees(lst1)) # Rad to Deg
lst2 = [90, 180]
print(numpy.radians(lst2)) # Deg to Rad
Output:
[ 90. 180.]
[1.57079633 3.14159265]
This module also has deg2rad()
and rad2deg()
functions used to perform the same function but have a more descriptive name.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn