How to Define Lists as Global Variable in Python
- Understanding Global Variables in Python
- Method 1: Defining a Global List Outside Functions
- Method 2: Modifying a Global List Inside Functions
- Method 3: Using Global Lists in Class Methods
- Conclusion
- FAQ
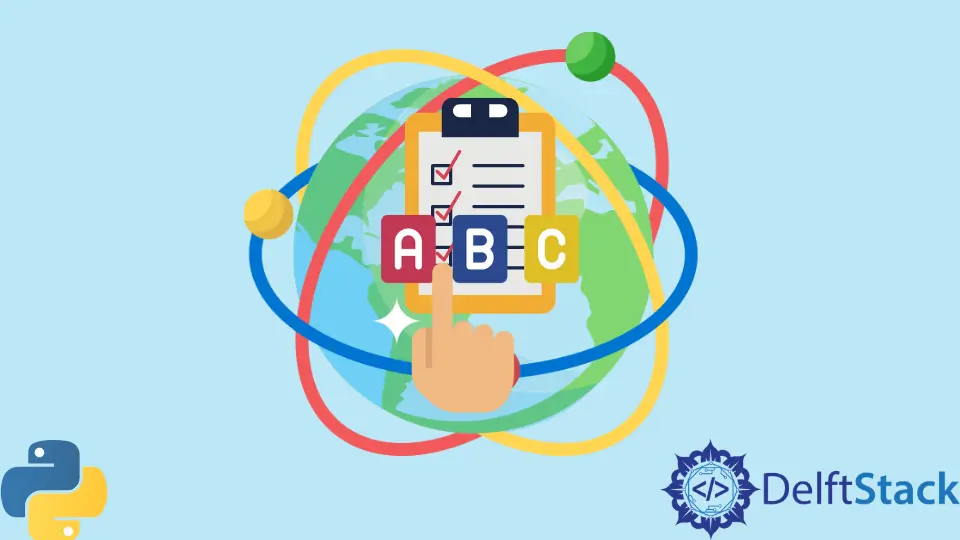
In the world of programming, understanding variable scope is crucial. One common requirement is to define a list as a global variable in Python. This allows you to access and modify the list from anywhere in your code, making it a powerful tool for managing data across different functions. Whether you’re working on a simple script or a complex application, knowing how to effectively utilize global variables can streamline your coding process.
In this tutorial, we’ll explore how to define lists as global variables in Python, providing clear examples and explanations to help you grasp the concept easily. Let’s dive in!
Understanding Global Variables in Python
Before we get into the specifics of defining lists as global variables, it’s essential to understand what global variables are. In Python, a global variable is one that is defined outside of any function and can be accessed throughout the entire program. This means that any function can read or modify the global variable, making it a shared resource.
When it comes to lists, defining them as global allows you to maintain a single instance of that list, which can be modified by various functions. This is particularly useful when you need to keep track of state or share data across different parts of your application without passing the list explicitly as an argument.
Let’s look at how to define a list as a global variable in Python.
Method 1: Defining a Global List Outside Functions
The most straightforward way to create a global list in Python is to define it at the top level of your script, outside any functions. This allows any function within that script to access and modify the list directly.
Here’s how you can do it:
my_list = []
def add_item(item):
global my_list
my_list.append(item)
def display_list():
print(my_list)
add_item('apple')
add_item('banana')
display_list()
Output:
['apple', 'banana']
In this example, we first define an empty list called my_list
outside of any functions. The add_item
function modifies this list by using the global
keyword, which tells Python that we want to use the global version of my_list
, not create a new local variable. After adding items to the list, we call display_list
, which prints the current contents of my_list
. This method is straightforward and effective for managing global lists.
Method 2: Modifying a Global List Inside Functions
Another approach to using global lists in Python is to modify them directly within functions without explicitly declaring them as global each time. However, this requires careful management to avoid scope-related issues.
Here’s an example:
my_list = ['item1', 'item2']
def modify_list():
my_list.append('item3')
def show_list():
print(my_list)
modify_list()
show_list()
Output:
['item1', 'item2', 'item3']
In this scenario, we have a global list initialized with two items. The modify_list
function appends a third item to my_list
. When we call show_list
, it prints the updated list. While this method works without the global
keyword, it’s crucial to ensure that no local variable with the same name exists within the function, as that would lead to confusion and potential errors.
Method 3: Using Global Lists in Class Methods
When working with classes, you might also want to define a list as a global variable accessible across different methods. This can be achieved by using class-level attributes, which can serve a similar purpose to global variables.
Here’s how you can implement this:
class MyClass:
my_list = []
@classmethod
def add_item(cls, item):
cls.my_list.append(item)
@classmethod
def show_list(cls):
print(cls.my_list)
MyClass.add_item('apple')
MyClass.add_item('banana')
MyClass.show_list()
Output:
['apple', 'banana']
In this example, my_list
is defined as a class-level attribute. The add_item
and show_list
methods are class methods, allowing them to access and modify my_list
directly. This approach is particularly useful when you want to encapsulate behavior related to the list within a class, maintaining a clean structure while still utilizing global-like data.
Conclusion
Defining lists as global variables in Python can significantly enhance your programming capabilities by allowing data to be shared across functions and classes. Whether you choose to define a global list outside of functions, modify it within functions, or use class-level attributes, understanding how to manage global variables effectively is key to writing efficient and organized code. With the examples provided, you should now have a solid foundation for implementing global lists in your own Python projects.
FAQ
-
what is a global variable in Python?
A global variable is defined outside any function and can be accessed from any part of the code. -
how do I modify a global list in a function?
Use theglobal
keyword inside the function to indicate that you want to modify the global list. -
can I use global lists in classes?
Yes, you can define a list as a class-level attribute to be accessed by class methods. -
what happens if I don’t use the global keyword?
If you try to assign a value to a global variable without using theglobal
keyword, Python will treat it as a local variable, leading to potential errors. -
is it a good practice to use global variables?
While global variables can be useful, overusing them can lead to code that is hard to understand and maintain. Always consider alternatives like function parameters or class attributes.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn