How to Decrement a Loop in Python
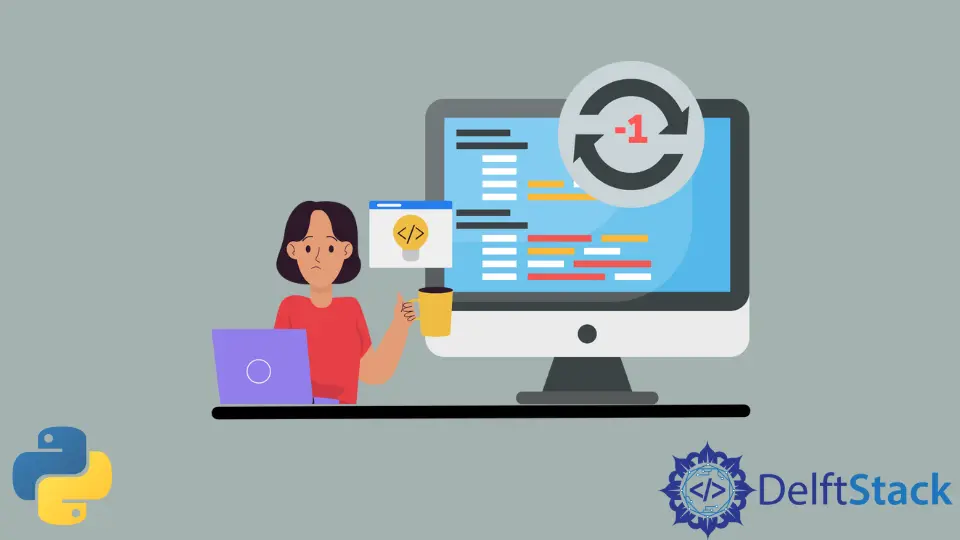
In Programming, a loop is a structure of instructions that iterates until a condition is True or a particular condition is met. In Python, we work with two types of loops, the For loop and the While loop.
Decrementing a loop means iterating the instructions backward in the loop. In this tutorial, we will decrement a loop in Python.
We generally use the range()
function with the For loop to create a series over which the loop iterates. The range()
function generates a series of numbers from the given starting point till the ending point. The start
and end
positions of this series can be specified using the start and stop parameters. The step
parameter determines the increment or decrement step value.
For decrementing the For loop, we use the step value as a negative integer.
For example,
for i in range(5, 0, -1):
print(i)
Output:
5
4
3
2
1
In the above example, the starting point is set as a higher limit and the endpoint as a lower limit, and a negative step value for decrementing for the loop.
We can also decrement a While loop. The While loop is used just like the for loop for a given set of statements until a given condition is false.
We assign a higher starting value to use in the condition in case of decrementing. A test condition is provided to run the loop till this condition is True. We decrement the value of the initial value in every iteration to decrement it.
For example,
i = 5
while i > 0:
print(i)
i = i - 1 # Decrementing
Output:
5
4
3
2
1