How to Declare a Variable Without Value in Python
-
Use the
None
Keyword to Declare a Variable Without Value in Python - Use the Variable Annotations to Declare a Variable Without Value in Python
- Use the Empty Strings or Lists to Declare a Variable Without Value in Python
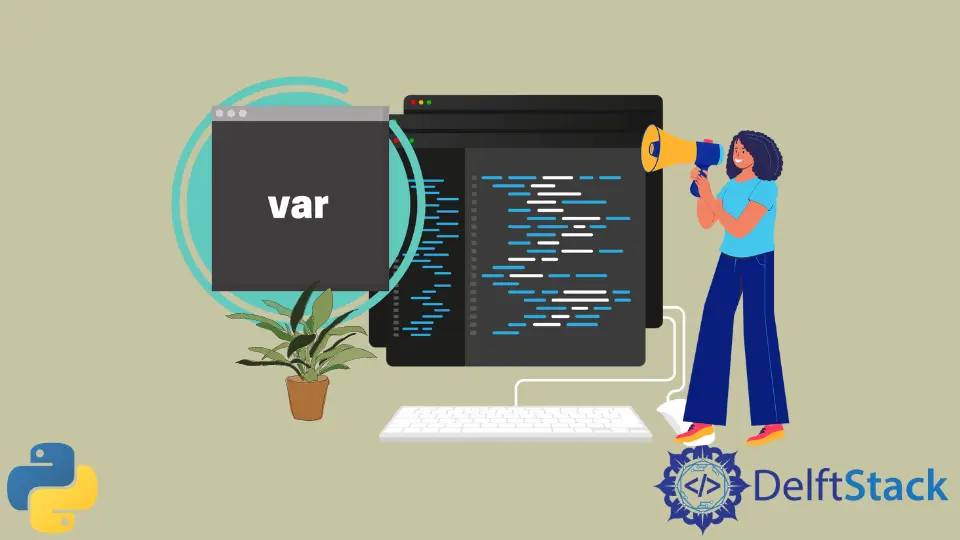
A variable is a reserved memory location that can store some values. In other words, a variable in a Python program gives data to the computer for processing the operations. Every value in Python has a data type. There are numbers, lists, tuples, and more in Python.
We will now discuss how to declare a variable without assigning it any value in Python.
Use the None
Keyword to Declare a Variable Without Value in Python
Python is dynamic, so one does not require to declare variables, and they exist automatically in the first scope where they are assigned. Only a regular assignment statement is required.
The None
is a special object of type NoneType
. It refers to a NULL value or some value that is not available. We can assign a variable as None
if we do not wish to give it any value.
For example,
var = None
This is convenient because one will never end up with an uninitialized variable. But this doesn’t mean that one would not end up with incorrectly initialized variables, so one should be careful.
Use the Variable Annotations to Declare a Variable Without Value in Python
For users with Python 3.6+, one may use the Variable Annotations for this situation.
Type Annotations were introduced in PEP 484. its main focus was function annotations. However, it also introduced the notion of type comments to annotate variables.
We can tell the type of the variable using this and not initialize it with any value.
The new PEP 526 introduced the syntax for annotating variables of the required type (including class variables and instance variables) without comments.
For example,
from typing import get_type_hints
var: str
It thus declares a variable named var
with no as such initial value.
Use the Empty Strings or Lists to Declare a Variable Without Value in Python
Apart from the methods discussed above, we can assign empty strings or lists to variables.
Technically, we assign a variable a value, but it is empty and updated according to our needs.
var = ""
lst = []