How to Declare a Dictionary in Python
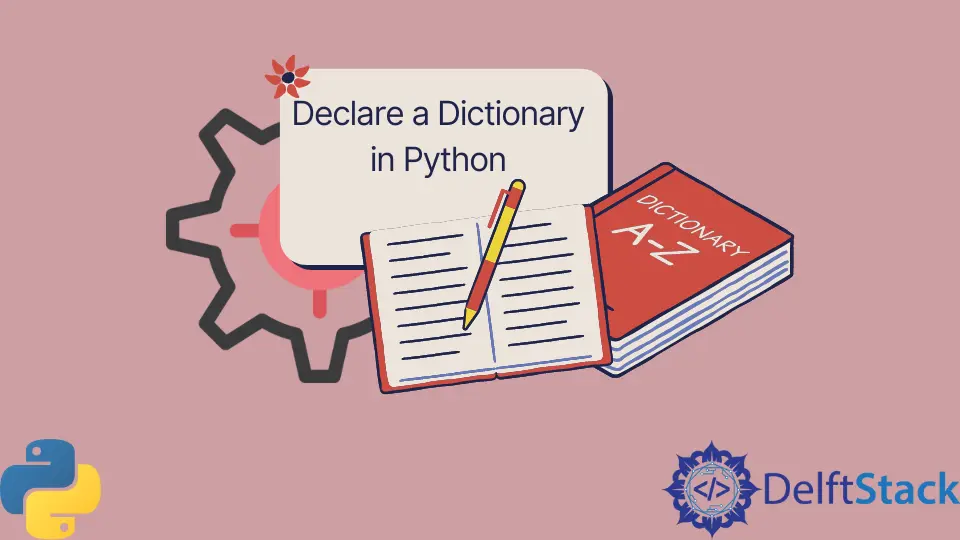
This tutorial will tackle the various methods to declare a dictionary data type in Python.
A dictionary in Python is a composite data type that can store data values as key:value
pairs. The data stored in a dictionary is unordered, mutable, and does not allow duplicates.
We can declare and store data in a dictionary in Python in the following ways.
Declare a Dictionary in Python Using {}
We can declare a dictionary data type in Python using {}
. We can either add the data as a key:value
pair before declaring a dictionary or add the data later.
Compared to using the dict()
constructor, using {}
is a much faster way to declare a dictionary. The following example code demonstrates how to declare and add data in a dictionary in Python.
mydict = {"name": "ali"}
mydict["age"] = 21
print(mydict)
Output:
{'name': 'ali', 'age': 21}
Declare a Dictionary in Python Using the dict()
Function
Although the {}
method is faster than using the dict()
constructor function, in cases where we do not have to declare a dictionary, again and again, the dict()
function is preferred as it is more readable. Also, it looks better to declare a dictionary.
Like in the {}
method, we can also add data in the dictionary while declaring it using the dict()
function. Unlike in the {}
method, the dict()
function does not take data input as key:value
pair, we pass the data as dict(key = value)
.
The following example shows how to utilize the dict()
function to declare and add data in a dictionary in Python.
mydict = dict(name="ali")
mydict["age"] = 21
print(mydict)
Output:
{'name': 'ali', 'age': 21}