How to Capture and Save Video Using OpenCV
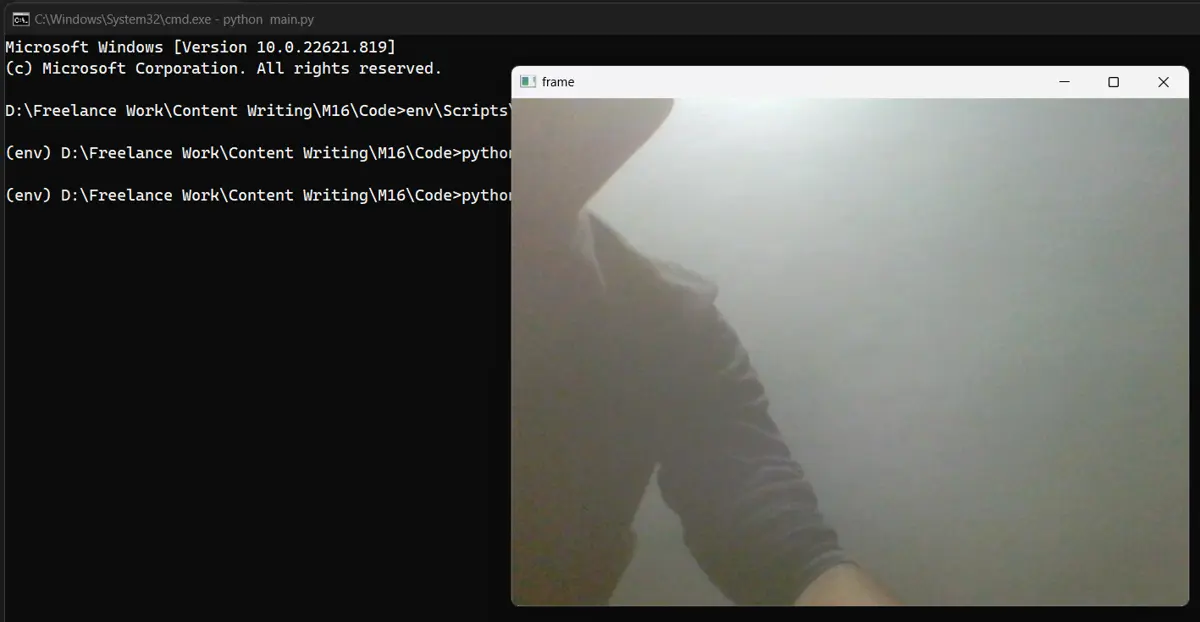
Computer Vision is a domain of Computer Science, specifically Artificial Intelligence, that lets computer systems understand and extract details from digital images, videos, and other visual sources.
Using computer vision, we can perform various complex tasks such as object detection, image classification, segmentation, edge detection, facial recognition, pattern detection, and feature matching.
Nowadays, various programming languages offer support for computer vision, including Python. Python is a versatile dynamically-typed general-purpose programming language.
It has a library, OpenCV or Open Computer Vision, that helps us perform computer vision.
OpenCV is a real-time computer vision library developed by Intel. It is a cross-platform library written in C and C++ programming languages.
This library lets us perform various tasks such as capturing video, processing video frames, manipulating colors and transforming images and videos.
In this article, we will learn how to record web camera video and save it using OpenCV.
Save Camera Video Using OpenCV
Refer to the following Python code.
import cv2
capture = cv2.VideoCapture(0)
fourcc = cv2.VideoWriter_fourcc(*"XVID")
out = cv2.VideoWriter("output.avi", fourcc, 20.0, (640, 480))
while capture.isOpened():
ret, frame = capture.read()
if ret == True:
out.write(frame)
cv2.imshow("frame", frame)
if cv2.waitKey(1) & 0xFF == ord("q"):
break
else:
break
capture.release()
out.release()
cv2.destroyAllWindows()
The Python code above records whatever the web camera of your system sees, and when the user presses the Q key on the keyboard, it stops recording, close the camera window, and saves the video file by the name of output.avi
in the working directory.
First, we use the cv2.VideoCapture()
method to get a video capture object for the system’s camera. Next, we specify the video codec using the cv2.VideoWriter_fourcc()
method.
Next, we declare the camera window size and output properties.
Next, we declare a for
loop that captures whatever the camera sees and saves it until Q key is pressed, and the loop ends. Lastly, the video is saved, the camera window is destroyed, and the web camera is released.