How to Crop Image Using OpenCV in Python
Manav Narula
Feb 02, 2024
Python
Python OpenCV
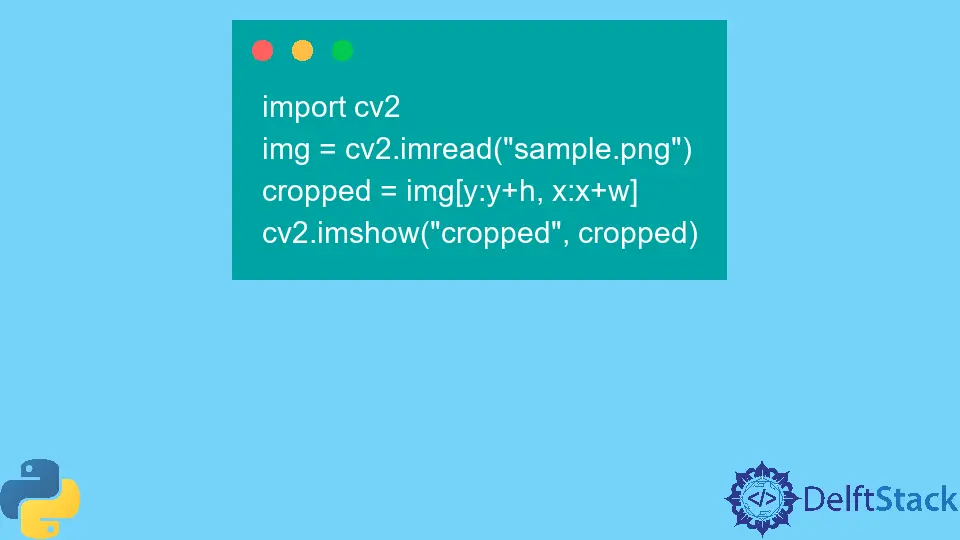
The opencv
module provides different tools and functionalities for Computer Vision. We have different functions available to read and process images.
This tutorial will demonstrate how to crop an image using the opencv
module in Python.
Images are stored as NumPy
arrays. To crop images, we can use NumPy
slicing to slice the arrays.
For example,
import cv2
img = cv2.imread("sample.png")
cropped = img[y : y + h, x : x + w]
cv2.imshow("cropped", cropped)
In the above example, the imread()
function reads the image. We crop the image using NumPy
slicing. Finally, we display the cropped image using the imshow()
function.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Manav Narula
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn