How to Create Package in Python
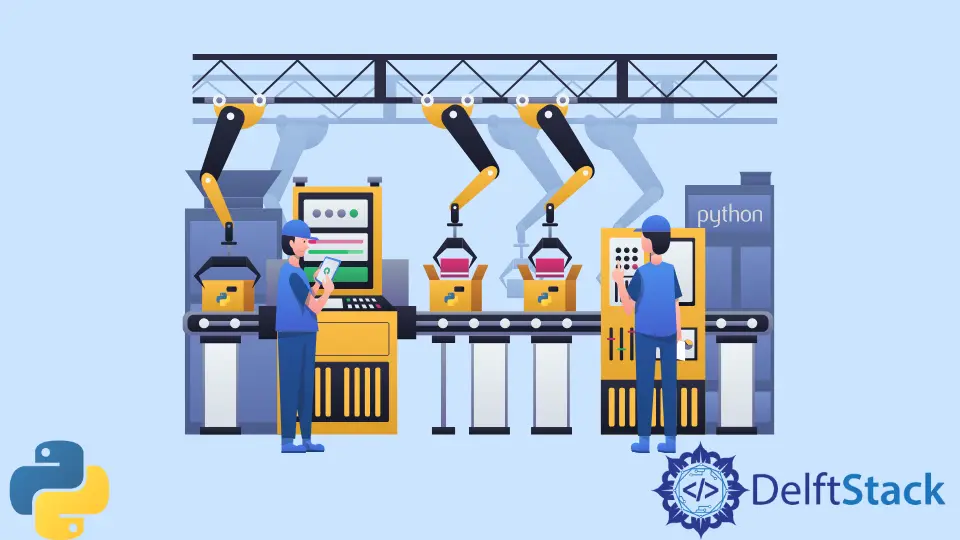
We will introduce how to create a package in Python.
Create Package in Python
Python is a widely-used programming language that can easily help us achieve many tasks. Python can be used for web development to software development.
In this article, we will study how to create a package in Python. A package is a reusable file of code that we can use for multiple purposes by importing the main file from the package and using the rest of the functions and definitions defined in those files.
Let’s create a new Python package with some functions, definitions, classes, and objects. First, we will create a new folder, pythonPrograms
.
In this folder, we will create another folder named mathFunctions
.
Once we have created our folders, we will let Python know that this is a package by creating an __init__.py
file. We will create sum
, subtract
, multiply
, and divide
modules.
First of all, we will create Sum.py
with the following code.
# python
class Sum:
def SumofNums(a, b):
print("Adding a and b, Answer:", a + b)
Similarly, we will create Subtract.py
with the following code.
# python
class Subtract:
def SubofNums(a, b):
print("Subtracting a and b, Answer:", a - b)
Similarly, we will create Multiply.py
with the following code.
# python
class Multiply:
def MultiplyofNums(a, b):
print("Multiplying a and b, Answer:", a * b)
Similarly, we will create Divide.py
with the following code.
# python
class Divide:
def DivideofNums(a, b):
print("Dividing a and b, Answer:", a / b)
Now, let’s create __init__.py
and add the following initialized code as shown below.
# python
from Sum import Sum
from Subtract import Subtract
from Multiply import Multiply
from Divide import Divide
In the pythonPrograms
folder, we will create a new file, sample.py
, and we will try to use these functions from our newly created mathFunctions
package, as shown below.
# python
from mathFunctions import Sum
from mathFunctions import Subtract
from mathFunctions import Multiply
from mathFunctions import Divide
a = 20
b = 2
# Adding a and b
Sum.SumofNums(a, b)
# Subtracting a and b
Subtract.SubofNums(a, b)
# Multiply a and b
Multiply.MultiplyofNums(a, b)
# Divide a and b
Divide.DivideofNums(a, b)
Output:
As seen from the above example, we can easily create packages for multiple functions or perform module tasks and reuse code as much as possible.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn