How to Create an Abstract Class in Python
-
Python 3.4+ - Create an Abstract Class in Python by Inheriting From the
ABC
Module -
Python 3.0+ - Create an Abstract Class in Python by Inheriting From the
ABCMeta
Module - Method to Verify if a Class Is Abstract or Not in Python
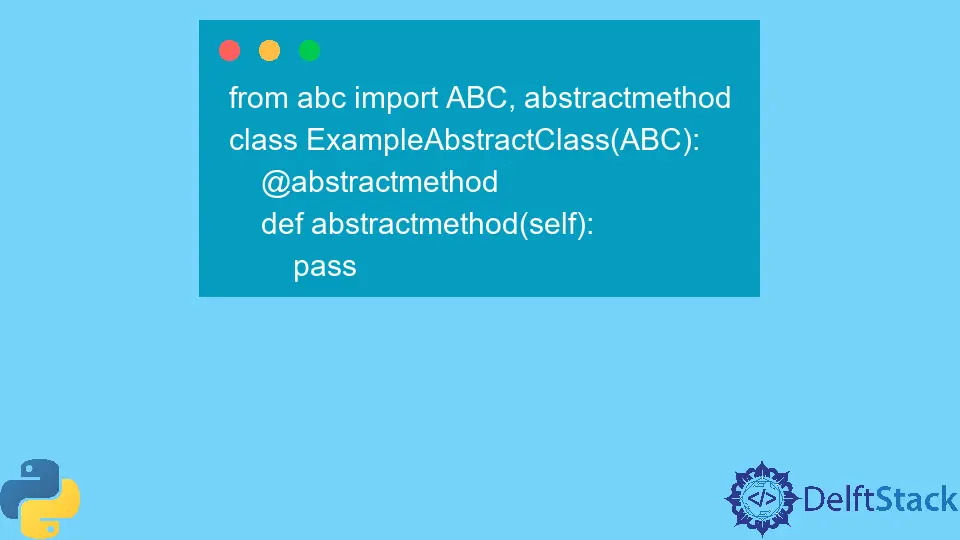
An abstract class is a restricted class because it cannot be instantiated - you cannot use it to create objects. It can only be inherited from another class.
An abstract class aims to define a common function/behaviour that multiple sub-classes can inherit without having to implement the entire abstract class.
In Python, we can create an abstract class-based in different ways based on the Python version.
Python 3.4+ - Create an Abstract Class in Python by Inheriting From the ABC
Module
In Python 3.4, to create an abstract class.
- Import the
ABC
(abstract base classes) module.
from abc import ABC, abstractmethod
- Declare the abstract method using the
@abstractmethod
decorator.
from abc import ABC, abstractmethod
class ExampleAbstractClass(ABC):
@abstractmethod
def abstractmethod(self):
pass
Below is an example where the Vehicle
abstract class has two subclasses, namely, Car
and Bike
. The classes Car
and Bike
have their unique implementation.
from abc import ABC, abstractmethod
class Vehicle(ABC):
@abstractmethod
def numberofwheels(self):
pass
class Car(Vehicle):
def numberofwheels(self):
print("A Car has 4 wheels")
class Bike(Vehicle):
def numberofwheels(self):
print("A Bike has 2 wheels")
C = Car()
C.numberofwheels()
B = Bike()
B.numberofwheels()
Output:
A Car has 4 wheels
A Bike has 2 wheels
Python 3.0+ - Create an Abstract Class in Python by Inheriting From the ABCMeta
Module
In Python 3.0+, to create an abstract class.
- Import the
ABCMeta
(abstract base classes) module.
from abc import ABCMeta, abstractmethod
- Declare the abstract method using the
@abstractmethod
decorator and mentionmetaclass=ABCMeta
.
from abc import ABCMeta, abstractmethod
class Example2AbstractClass(metaclass=ABCMeta):
@abstractmethod
def abstractmethod2(self):
pass
Below is an example.
from abc import ABCMeta, abstractmethod
class Vehicle(metaclass=ABCMeta):
@abstractmethod
def numberofwheels(self):
pass
class Car(Vehicle):
def numberofwheels(self):
print("A Car has 4 wheels")
class Bike(Vehicle):
def numberofwheels(self):
print("A Bike has 2 wheels")
C = Car()
C.numberofwheels()
B = Bike()
B.numberofwheels()
Output:
A Car has 4 wheels
A Bike has 2 wheels
Method to Verify if a Class Is Abstract or Not in Python
To verify if the class created is indeed an abstract class or not, we should instantiate the class. An abstract class will not allow instantiation and throw an error.
For example, if we instantiate an abstract as below.
x = ExampleAbstractClass()
Then, an error is displayed.
Can't instantiate abstract class ExampleAbstractClass with abstract methods abstractmethod