How to Create a Dice Roll Simulator in Python
Najwa Riyaz
Feb 02, 2024
Python
Python Number
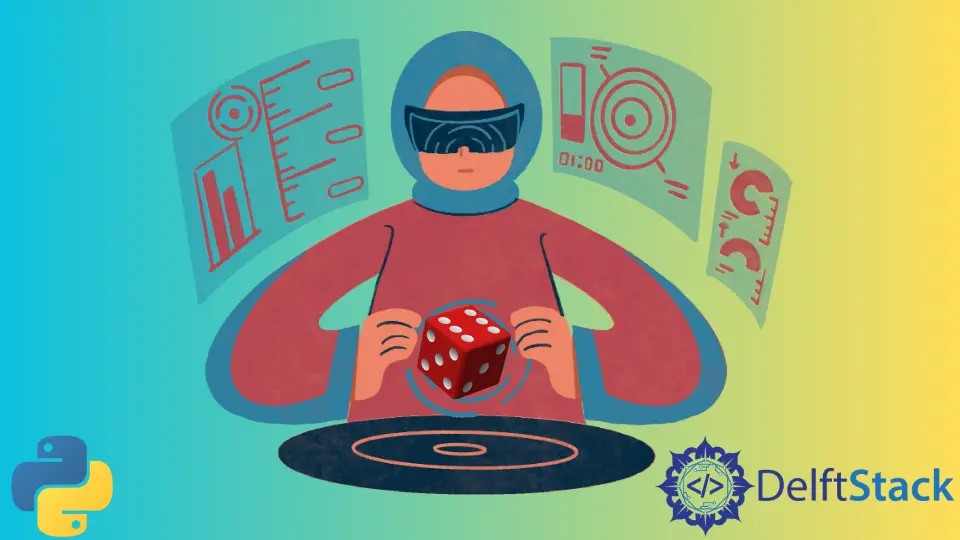
To create a dice roll simulator in Python, we use the random.randint()
function generates random numbers between the range of numbers 1 to 6 as follows.
random.randint(1, 6)
Create a Dice Roll Simulator in Python Using random.randint(1,6)
We can create a dice roll simulator in Python using the random.randint()
function. The syntax of the function is as follows.
random.randint(x, y)
Accordingly, it generates a random integer between x
and y
. In the dice simulator example,
x
is 1, and y
is 6.
Below is an example.
import random
print("You rolled the following number", random.randint(1, 6))
To let the user choose whether to continue rolling the dice or not, we can place random.randint(1,6)
within a while
loop as follows.
from random import randint
repeat_rolling = True
while repeat_rolling:
print("You rolled the following number using the Dice -", randint(1, 6))
print("Do you wish to roll the dice again?")
repeat_rolling = ("y" or "yes") in input().lower()
When the user chooses to stop rolling the dice, it should exit the while
loop.
Output:
You rolled the following number using the Dice - 2
Do you wish to roll the dice again?
y
You rolled the following number using the Dice - 4
Do you wish to roll the dice again?
y
You rolled the following number using the Dice - 5
Do you wish to roll the dice again?
n
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe