How to Count Vowels in a String Using Python
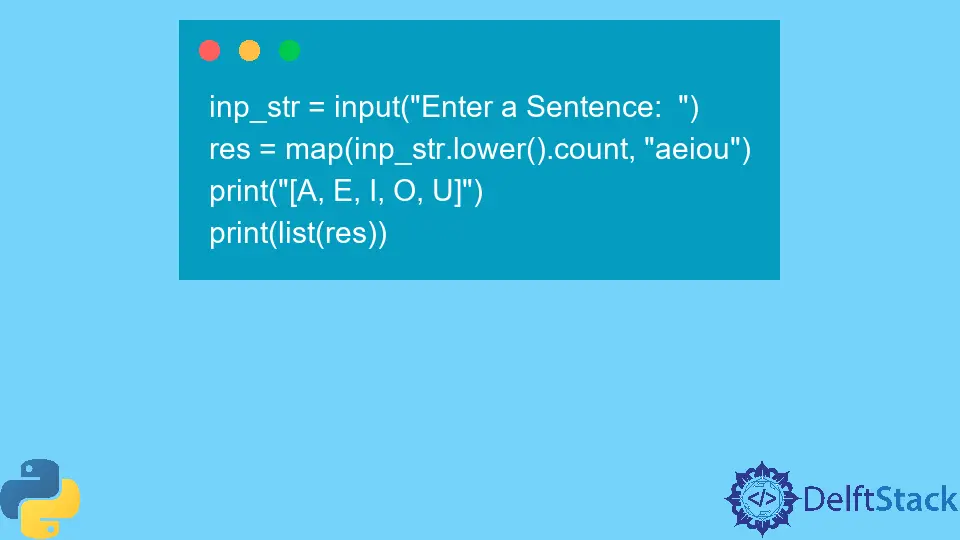
This brief programming tutorial is a guide on getting a count of vowels from a string using Python.
Count Vowels in a String Using Python
Python provides us with many functions that can be used to count the number of characters from a string. These functions can be combined to count the number of vowels from the string.
Let’s look at the following code snippet.
inp_str = input("Enter a Sentence: ")
res = map(inp_str.lower().count, "aeiou")
print("[A, E, I, O, U]")
print(list(res))
In this code snippet, we first took a string from the user as input and stored it in a variable inp_str
. Then, we used a function map that expects two arguments: the name of a function and the list object (iterable).
It then applies the passed function (i.e., count
) to the inp_str
list for each character in the aeiou
list.
For example, in the above code, we have passed the count
function and a list of vowels (i.e., aeiou
). The map()
function will now count each vowel in the inp_str
separately and saves its count in the res
object.
In the end, the res
object will also be an iterable object containing the count of each vowel at its indexes. Let’s look at the output of this code.
Line 3 of the code is just for the sake of increasing the readability of the output so that we can easily figure out the count of each vowel of the string.
Note that before counting the vowels, we have made the string lowercase by calling the function lower()
to avoid the hassle of case sensitivity.