How to Convert Tuple to List in Python
-
Use the
list()
Function to Convert a Tuple to a List in Python -
Use the Unpack Operator
*
to Convert a Tuple to a List in Python - Use the List Comprehension Method to Convert a Tuple to a List in Python
-
Use the
map()
Function to Convert a Tuple to a List in Python
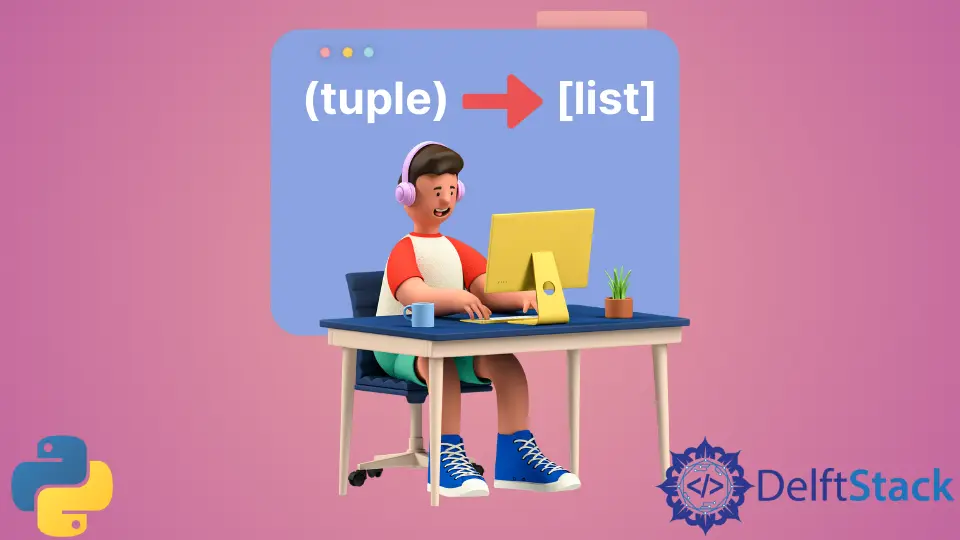
A tuple is a built-in data type that is used to store multiple values in a single variable. It is written with round brackets and is ordered and unchangeable.
A list is a dynamically sized array that can be both homogeneous and heterogeneous. It is ordered, has a definite count, and is mutable, i.e., and we can alter it even after its creation.
We convert a tuple into a list using this method.
Use the list()
Function to Convert a Tuple to a List in Python
The list()
function is used to typecast a sequence to a list and initiate lists in Python.
We can use it to convert a tuple to a list.
For Example,
tup1 = (111, "alpha", "beta", "gamma", 222)
list1 = list(tup1)
print("list elements are:", list1)
Output:
list elements are: [111,'alpha','beta','gamma',222]
Here, the entered tuple in tup1
has been converted into a list list1
.
Use the Unpack Operator *
to Convert a Tuple to a List in Python
The *
operator to unpack elements from an iterable. It is present in Python 3.5 and above. We can use it to convert a tuple to a list in Python.
For example,
tup1 = (111, "alpha", "beta", "gamma", 222)
list1 = [*tup1]
print("list elements are:", list1)
Output:
list elements are: [111,'alpha','beta','gamma',222]
Use the List Comprehension Method to Convert a Tuple to a List in Python
List comprehension is an elegant, concise way to create lists in Python with a single line of code. We can use this method to convert a tuple containing multiple tuples to a nested list.
See the following example.
tup1 = ((5, 6, 8, 9), (9, 5, 4, 2))
lst = [list(row) for row in tup1]
print(lst)
Output:
[[5, 6, 8, 9], [9, 5, 4, 2]]
Use the map()
Function to Convert a Tuple to a List in Python
The map()
function can apply a function to each item in an iterable. We can use it with the list()
function to convert tuple containing tuples to a nested list.
For example,
tup1 = ((5, 6, 8, 9), (9, 5, 4, 2))
lst = list(map(list, tup1))
print(lst)
Output:
[[5, 6, 8, 9], [9, 5, 4, 2]]
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python