How to Convert Date to Datetime in Python
- Understanding the Date and Datetime Objects
- Method 1: Using the combine Function
- Method 2: Using the replace Method
- Method 3: Using the strptime Function
- Conclusion
- FAQ
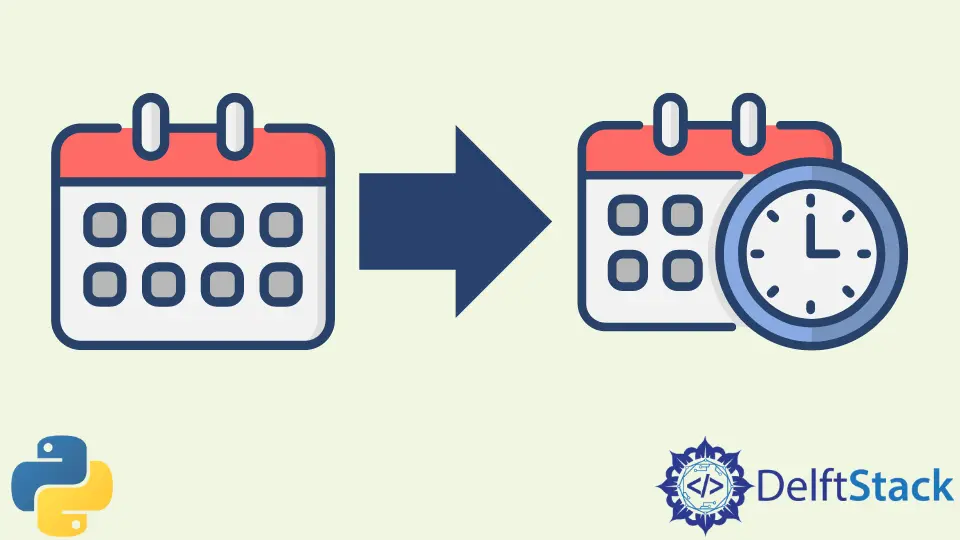
Converting a date to a datetime object in Python is a common task that many developers encounter. Whether you’re dealing with data analysis, web development, or any project that requires precise time manipulation, understanding how to perform this conversion is essential.
In this tutorial, we’ll explore how to effectively convert date objects into datetime objects using Python’s built-in datetime
module. We’ll cover various methods and provide clear code examples to illustrate each approach. By the end of this article, you will have a strong grasp of how to handle date and datetime conversions in Python, making your coding journey smoother and more efficient.
Understanding the Date and Datetime Objects
Before diving into the conversion methods, it’s important to understand what date and datetime objects are in Python. The date
object represents a calendar date (year, month, day) without any time component, while the datetime
object contains both date and time information (year, month, day, hour, minute, second, and microsecond). This distinction is crucial when performing conversions, as it determines how the data is handled and manipulated.
The datetime
module provides various classes, including date
, time
, and datetime
, which can be used to create and manipulate date and time information. To convert a date object to a datetime object, you typically want to append a specific time to the date. Let’s explore how to do this using different methods.
Method 1: Using the combine Function
One of the simplest ways to convert a date to a datetime object is by using the combine
function from the datetime
module. This method allows you to merge a date object with a time object to create a datetime object.
from datetime import date, time, datetime
# Create a date object
d = date(2023, 10, 15)
# Create a time object
t = time(14, 30)
# Combine date and time
dt = datetime.combine(d, t)
print(dt)
Output:
2023-10-15 14:30:00
In this example, we first import the necessary classes from the datetime
module. We then create a date
object representing October 15, 2023, and a time
object representing 2:30 PM. The combine
function merges these two objects into a single datetime
object. The resulting output shows the complete date and time.
This method is particularly useful when you have a specific time you want to associate with the date. It gives you full control over both components, allowing for precise datetime creation.
Method 2: Using the replace Method
Another effective way to convert a date to a datetime object is by using the replace
method. This method allows you to modify an existing date object by adding time information directly.
from datetime import date
# Create a date object
d = date(2023, 10, 15)
# Convert date to datetime by replacing the time
dt = d.replace(hour=14, minute=30)
print(dt)
Output:
2023-10-15 14:30:00
In this example, we create a date
object for October 15, 2023, just like before. However, instead of creating a separate time object, we use the replace
method to specify the hour and minute directly on the date object. The output shows the combined date and time as a datetime
object.
This method is advantageous when you want to quickly set a time without needing to create a separate time object. It’s a straightforward approach that keeps your code concise and readable.
Method 3: Using the strptime Function
If you have a date represented as a string, you can convert it to a datetime object using the strptime
function. This method is particularly useful when dealing with date formats in string form.
from datetime import datetime
# Date string
date_string = "2023-10-15"
# Convert string to datetime
dt = datetime.strptime(date_string, "%Y-%m-%d")
print(dt)
Output:
2023-10-15 00:00:00
In this example, we have a date in string format. By using the strptime
method, we can parse this string into a datetime
object. The format string "%Y-%m-%d"
tells Python how to interpret the date components. The output shows the date at midnight since no specific time was provided in the string.
Using strptime
is incredibly useful for data input scenarios where dates are often received as strings, especially in web applications or data processing tasks. It allows for flexible parsing of various date formats.
Conclusion
In this tutorial, we’ve explored three effective methods to convert a date to a datetime object in Python: using the combine
function, the replace
method, and the strptime
function. Each method serves different use cases, from merging date and time components to parsing date strings. Understanding these techniques will enhance your ability to manipulate date and time data in your Python projects. With these skills, you can confidently handle various datetime scenarios, making your coding experience more efficient and enjoyable.
FAQ
-
What is the difference between date and datetime in Python?
Thedate
object represents a calendar date without time, while thedatetime
object includes both date and time components. -
Can I convert a datetime object back to a date object?
Yes, you can use thedate()
method on a datetime object to extract the date component. -
What formats can I use with strptime?
You can use various format codes such as %Y for year, %m for month, and %d for day to match the string format. -
Is it necessary to specify time when converting date to datetime?
No, if you don’t specify time, it defaults to midnight (00:00:00). -
Can I use timezone-aware datetime objects?
Yes, you can create timezone-aware datetime objects using thepytz
library or the built-intimezone
class.
Abdul is a software engineer with an architect background and a passion for full-stack web development with eight years of professional experience in analysis, design, development, implementation, performance tuning, and implementation of business applications.
LinkedIn