How to Compare Lists in Python
-
Use the
for
Loop to Compare Lists in Python -
Use the
set
to Compare Lists in Python -
Use the
collection.counter()
Function to Compare Two Lists in Python
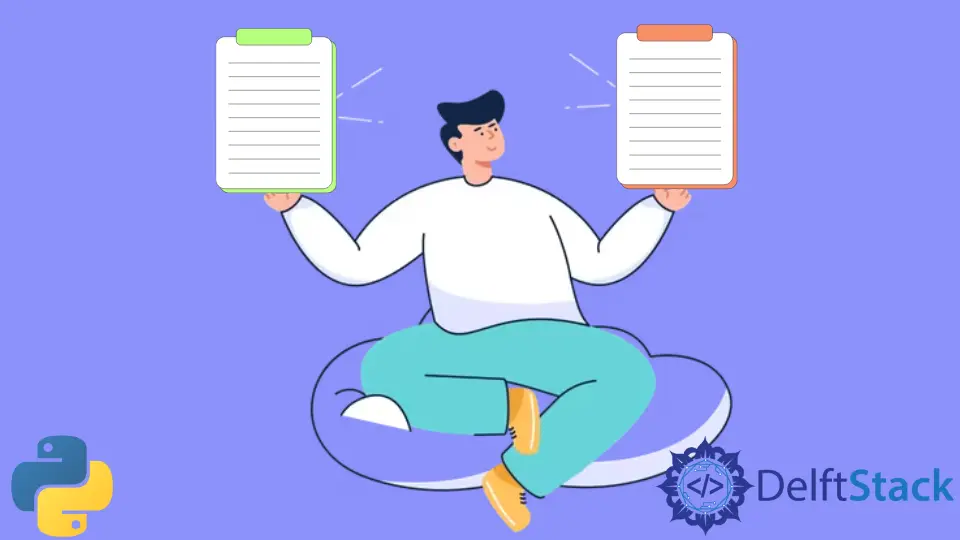
Lists are used to store multiple elements in a specific order in Python.
In this tutorial, we will learn how to compare two lists and find the elements that are equal.
Use the for
Loop to Compare Lists in Python
The for
loop can be used to iterate over the list and compare each element of one list with all the second list elements. With this method, we can find compare elements individually and find out the common elements.
The following code shows how to implement this.
l1 = [1, 2, 3]
l2 = [3, 2, 5]
for i in l1:
for j in l2:
if i == j:
print(i)
break
Output:
2
3
Use the set
to Compare Lists in Python
The Set
is a collection of elements and is unordered. We can directly convert a list to a set using the set()
function and compare them for equality.
For example,
l1 = [1, 2, 3, 4, 5]
l2 = [9, 8, 7, 6, 5]
if set(l1) == set(l2):
print("Lists are equal")
else:
print("Lists are not equal")
Output:
Lists are not equal
We can also find out the common elements between two lists using the &
operator, which returns the intersection of two sets.
For example,
l1 = [1, 2, 3, 4, 5]
l2 = [9, 8, 7, 6, 5]
print("Common Elements", set(l1) & set(l2))
Output:
Common Elements {5}
Use the collection.counter()
Function to Compare Two Lists in Python
The counter()
function returns a dictionary that contains all the elements in the list and their frequency as key-value pairs. We can compare this dictionary to check for equality and more.
For example,
import collections
l1 = [1, 2, 3]
l2 = [3, 2, 1]
if collections.Counter(l1) == collections.Counter(l2):
print("Equal")
else:
print("Not Equal")
Output:
Equal
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python