Colors in Python
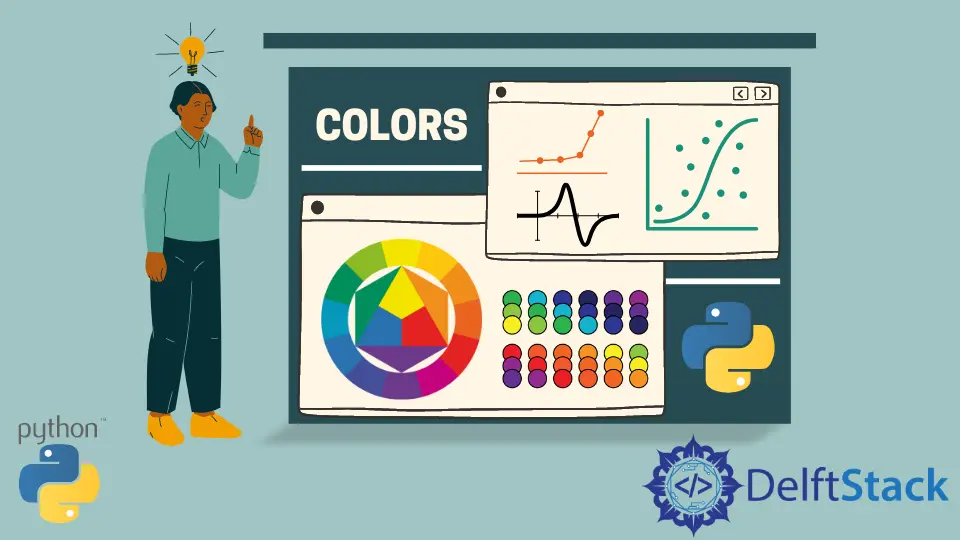
This article aims to introduce the named colors used by the Matplotlib
module in Python for displaying graphs. In plotting graphs, Python offers the option for users to choose named colors shown through its Matplotlib
library.
In Python, the color names and their hexadecimal codes are retrieved from a dictionary in the color.py
module.
In the following code, we print the names of the colors in this module.
import matplotlib
for cname, hex in matplotlib.colors.cnames.items():
print(cname, hex)
Output:
'aliceblue': '#F0F8FF',
'antiquewhite': '#FAEBD7',
'aqua': '#00FFFF',
'aquamarine': '#7FFFD4',
'azure': '#F0FFFF',
'beige': '#F5F5DC',
'bisque': '#FFE4C4',
'black': '#000000',
'blanchedalmond': '#FFEBCD',
'blue': '#0000FF',
'blueviolet': '#8A2BE2',
'brown': '#A52A2A',
'burlywood': '#DEB887',
'cadetblue': '#5F9EA0',
...more
The matplotlib.colors.cnames.items()
returns the collection of dictionaries where the color’s names and codes are stored. The name is stored as the key of the dictionary and the hexadecimal code as its value.
We can use these colors with different types of graphs and figures with compatible libraries. We can plot all these colors in a single graph and compare each color.
See the following code.
import matplotlib.pyplot as plt
import matplotlib.patches as patches
import matplotlib.colors as colors
import math
fig = plt.figure()
ax = fig.add_subplot(111)
ratio = 1.0 / 3.0
count = math.ceil(math.sqrt(len(colors.cnames)))
x_count = count * ratio
y_count = count / ratio
x = 0
y = 0
w = 1 / x_count
h = 1 / y_count
for cl in colors.cnames:
pos = (x / x_count, y / y_count)
ax.add_patch(patches.Rectangle(pos, w, h, color=cl))
ax.annotate(cl, xy=pos)
if y >= y_count - 1:
x += 1
y = 0
else:
y += 1
plt.show()
Output plot:
In the above code, we plotted each color with its respective name. We divided the figure into several subplots and plotted each color in a small rectangular patch using the add_patch()
function on each ax for each color.